RecyclerView背景平移
时间: 2023-08-28 17:06:51 浏览: 125
您好!感谢您的提问。根据您的问题描述,您需要实现RecyclerView的背景平移效果。下面是一个简单的实现思路:
1. 首先,您需要在RecyclerView的Adapter中记录当前选中的项的位置。
2. 在RecyclerView的ItemDecoration中,您可以通过重写onDraw()方法来绘制背景。
3. 在绘制背景时,您可以通过计算当前项与选中项的偏移量,来实现背景的平移效果。
4. 您可以使用ValueAnimator来实现背景平移的动画效果,并在选中项发生变化时启动动画。
5. 最后,根据您的需求,您可能还需要添加一些边界处理代码,来确保背景不会超出RecyclerView的边界。
下面是一个简单的示例代码,仅供参考:
``` kotlin
class MyItemDecoration(private val context: Context) : RecyclerView.ItemDecoration() {
private var selectedPosition = RecyclerView.NO_POSITION
fun setSelectedPosition(position: Int) {
selectedPosition = position
}
override fun onDraw(c: Canvas, parent: RecyclerView, state: RecyclerView.State) {
super.onDraw(c, parent, state)
if (selectedPosition == RecyclerView.NO_POSITION) {
return
}
val selectedView = parent.getChildAt(selectedPosition) ?: return
val selectedViewTop = selectedView.top
val selectedViewBottom = selectedView.bottom
val selectedViewHeight = selectedView.height
val selectedViewCenterY = selectedViewTop + selectedViewHeight / 2
val recyclerViewHeight = parent.height
val halfRecyclerViewHeight = recyclerViewHeight / 2
val translationY = halfRecyclerViewHeight - selectedViewCenterY
c.save()
c.translate(0f, translationY.toFloat())
val background = ContextCompat.getDrawable(context, R.drawable.selected_background)
background?.setBounds(0, selectedViewTop, parent.width, selectedViewBottom)
background?.draw(c)
c.restore()
}
fun startAnimation(parent: RecyclerView) {
val view = parent.getChildAt(selectedPosition) ?: return
val translationY = view.translationY
val animator = ValueAnimator.ofFloat(translationY, 0f)
animator.addUpdateListener { valueAnimator ->
val value = valueAnimator.animatedValue as Float
view.translationY = value
}
animator.duration = 300
animator.start()
}
}
```
在您的Activity或Fragment中,您需要初始化MyItemDecoration,并将其添加到RecyclerView中:
``` kotlin
val itemDecoration = MyItemDecoration(context)
recyclerView.addItemDecoration(itemDecoration)
```
在您的Adapter中,您需要实现选中项的处理,在选中项发生变化时,您需要更新MyItemDecoration中的selectedPosition,并启动平移动画:
``` kotlin
class MyAdapter(private val context: Context) : RecyclerView.Adapter<MyViewHolder>() {
private var selectedPosition = RecyclerView.NO_POSITION
private val itemDecoration = MyItemDecoration(context)
fun setSelectedPosition(position: Int) {
val oldPosition = selectedPosition
selectedPosition = position
if (oldPosition != RecyclerView.NO_POSITION) {
notifyItemChanged(oldPosition)
}
if (position != RecyclerView.NO_POSITION) {
notifyItemChanged(position)
itemDecoration.setSelectedPosition(position)
itemDecoration.startAnimation(recyclerView)
}
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): MyViewHolder {
// ...
}
override fun onBindViewHolder(holder: MyViewHolder, position: Int) {
// ...
if (position == selectedPosition) {
holder.itemView.isSelected = true
} else {
holder.itemView.isSelected = false
}
}
override fun getItemCount() = dataList.size
}
```
这样,当选中项发生变化时,就会触发背景平移的动画效果。希望这些代码对您有所帮助!
阅读全文
相关推荐
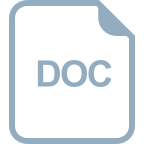
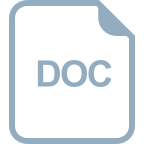
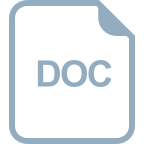












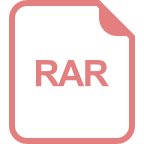
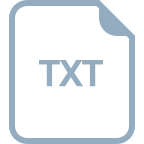
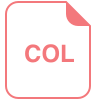
