用select处理RS485超时问题
时间: 2023-08-10 22:19:18 浏览: 266
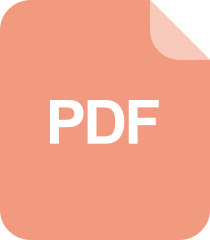
RS485应用中的问题
在使用RS-485进行通信时,由于通信距离较远,可能会发生信号传输延迟或丢失的情况,从而导致超时问题。为了解决这个问题,可以使用select函数来设置超时时间。
select函数是一种多路复用的I/O模型,它可以同时监视多个文件描述符,等待其中任意一个文件描述符就绪或超时。在使用RS-485进行通信时,可以将串口文件描述符添加到select监视列表中,并设置超时时间,当select函数返回时,检查串口文件描述符是否就绪,如果就绪则进行数据读写,否则进行超时处理。
以下是一个简单的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <sys/select.h>
int main()
{
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NONBLOCK);
if (fd < 0) {
perror("open ttyS0 failed");
return -1;
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &options);
fd_set rfds;
struct timeval tv;
int ret;
while (1) {
FD_ZERO(&rfds);
FD_SET(fd, &rfds);
tv.tv_sec = 1;
tv.tv_usec = 0;
ret = select(fd+1, &rfds, NULL, NULL, &tv);
if (ret < 0) {
perror("select failed");
break;
} else if (ret == 0) {
printf("timeout\n");
} else {
if (FD_ISSET(fd, &rfds)) {
char buf[1024];
int len = read(fd, buf, sizeof(buf));
if (len < 0) {
perror("read failed");
break;
} else if (len == 0) {
printf("EOF\n");
break;
} else {
// 处理接收到的数据
}
}
}
}
close(fd);
return 0;
}
```
上述代码中,首先打开串口设备文件/dev/ttyS0,并设置串口参数。然后在循环中使用select函数等待串口数据就绪或超时,当串口数据就绪时,使用read函数读取数据并进行处理。如果select函数返回超时,则进行超时处理。
阅读全文
相关推荐
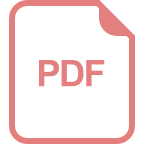
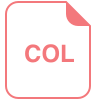
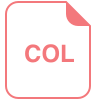


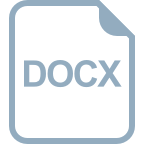
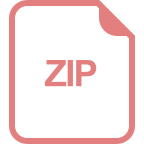
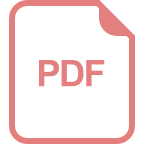
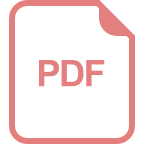
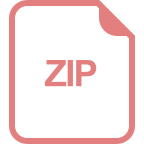
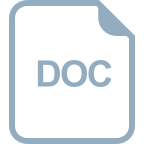





