给出相应的C语言代码
时间: 2024-11-28 20:13:03 浏览: 6
为了给出寻找两个有序链表交集并合并到原链表A中的C语言代码示例,我们可以使用结构体表示链表节点,并编写函数来完成这个任务。假设链表节点有三个字段:`val`存储节点值,`next`指向下一个节点,`prev`指向前一个节点(用于插入新节点时保持链表连续)。以下是代码片段:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode* next;
struct ListNode* prev;
} ListNode;
ListNode* getIntersectionNode(ListNode* headA, ListNode* headB) {
ListNode* p1 = headA;
ListNode* q1 = headB;
while (p1 != NULL && q1 != NULL) {
if (p1 == q1) {
return p1; // 找到了交点
}
if (p1->val < q1->val) {
p1 = p1->next; // A中较小的继续找
} else {
q1 = q1->next; // B中较小的继续找
}
}
return NULL; // 没有交集
}
void insertAndMergeIntersectionToList(ListNode** headA, ListNode* intersection) {
if (intersection == NULL || *headA == NULL) {
return; // 如果没有交集或链表为空,直接返回
}
// 将交点插到链表A的适当位置
ListNode* current = *headA;
ListNode* prev = NULL;
while (current != NULL && current->val < intersection->val) {
prev = current;
current = current->next;
}
intersection->prev = prev;
if (prev != NULL) {
prev->next = intersection;
} else {
*headA = intersection;
}
intersection->next = current;
// 连接交点后的部分
if (current != NULL) {
current->prev = intersection;
}
}
int main() {
// 假设已经有一个创建链表的例子
ListNode* headA = ...; // 链表A
ListNode* headB = ...; // 链表B
ListNode* intersection = getIntersectionNode(headA, headB);
if (intersection != NULL) {
insertAndMergeIntersectionToList(&headA, intersection); // 插入并连接
}
// 输出链表A以检查结果
ListNode* temp = headA;
while (temp != NULL) {
printf("%d ", temp->val);
temp = temp->next;
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
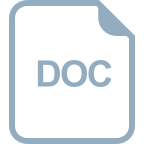
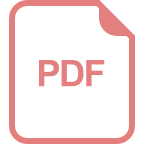
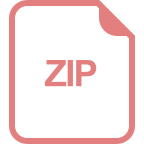
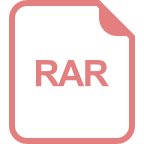
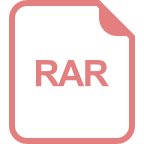
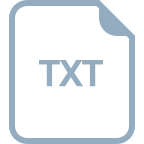
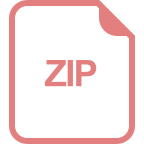
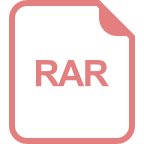
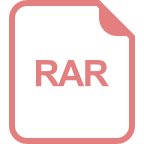
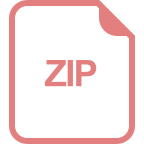
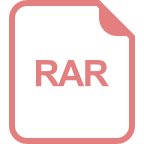
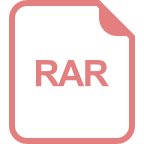
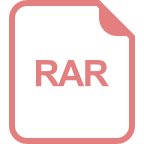
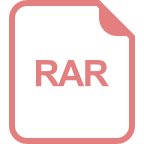
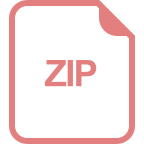
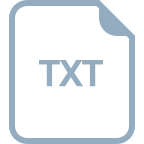
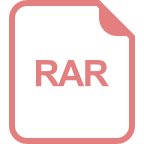