用Java一个数组a[4]里面有数据{1,2,3,4},另外一个数组b[3]里面有数据{5,6,7},将2个数组合并成一个数组c[7],并且打印出所有值
时间: 2024-02-25 22:58:38 浏览: 58
好的,我可以帮你解决这个问题。以下是 Java 代码示例:
```java
int[] a = {1, 2, 3, 4};
int[] b = {5, 6, 7};
int[] c = new int[a.length + b.length];
System.arraycopy(a, 0, c, 0, a.length);
System.arraycopy(b, 0, c, a.length, b.length);
for (int i = 0; i < c.length; i++) {
System.out.println(c[i]);
}
```
首先,我们定义了两个数组 `a` 和 `b`,分别赋值为 `{1, 2, 3, 4}` 和 `{5, 6, 7}`。然后,我们创建了一个新的数组 `c`,它的长度为 `a` 和 `b` 的长度之和。
接着,我们使用 `System.arraycopy()` 方法将数组 `a` 和 `b` 的元素复制到数组 `c` 中,其中第一个参数为源数组,第二个参数为源数组的起始位置,第三个参数为目标数组,第四个参数为目标数组的起始位置,第五个参数为要复制的元素数量。
最后,我们使用一个循环遍历数组 `c` 中的所有元素,并使用 `System.out.println()` 方法将它们打印出来。
希望这可以回答你的问题。
相关问题
用java写一个数组a[4]里面有数据{1,2,3,4},另外一个数组b[3]里面有数据{5,6,7},将2个数组合并成一个数组c[7],并且打印出所有值
可以使用Java中的System.arraycopy()方法实现两个数组合并成一个数组的操作,具体代码如下:
```java
public class MergeArrays {
public static void main(String[] args) {
int[] a = {1, 2, 3, 4};
int[] b = {5, 6, 7};
int[] c = new int[a.length + b.length];
// 将数组a复制到数组c中
System.arraycopy(a, 0, c, 0, a.length);
// 将数组b复制到数组c中
System.arraycopy(b, 0, c, a.length, b.length);
// 打印数组c中的所有值
for (int i = 0; i < c.length; i++) {
System.out.print(c[i] + " ");
}
}
}
```
输出结果为:
```
1 2 3 4 5 6 7
```
用java实现一个遍历一个数组A,将遍历出来的数据再装到另一个数组B中,但是数组B只能存三个数组,当B数组满三个后就自动创建新的数组C再往数组C里添加三个数组,让前一个数组满了后会创建另一个数组接着存储,最后将遍历出来存储的数组全部都存储在一个集合里面
可以使用Java中的ArrayList来实现这个功能。以下是一个示例代码:
```java
import java.util.ArrayList;
public class ArrayTraversal {
public static void main(String[] args) {
int[] arrayA = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; // 假设有一个数组A
ArrayList<int[]> collection = new ArrayList<>(); // 创建一个集合存储数组
int[] currentArray = new int[3]; // 创建当前数组
int currentIndex = 0; // 当前数组的索引
for (int i = 0; i < arrayA.length; i++) {
currentArray[currentIndex] = arrayA[i]; // 将遍历出来的数据存入当前数组
currentIndex++; // 当前数组索引加一
if (currentIndex == 3) { // 当当前数组满三个元素时
collection.add(currentArray); // 将当前数组存入集合
currentArray = new int[3]; // 创建新的数组
currentIndex = 0; // 重置当前数组的索引
}
}
// 处理最后一个数组(可能不满三个元素)
if (currentIndex > 0) {
int[] lastArray = new int[currentIndex];
System.arraycopy(currentArray, 0, lastArray, 0, currentIndex);
collection.add(lastArray);
}
// 打印集合中的数组
for (int[] array : collection) {
System.out.print("[");
for (int i = 0; i < array.length; i++) {
System.out.print(array[i]);
if (i != array.length - 1) {
System.out.print(", ");
}
}
System.out.println("]");
}
}
}
```
这个示例代码中,首先创建了一个数组A,然后使用ArrayList来存储数组。遍历数组A,将数据存入当前数组currentArray,并在满足条件时将当前数组存入集合collection。最后,打印出集合中的所有数组。请注意,最后一个数组可能不满三个元素,需要特别处理一下。
阅读全文
相关推荐
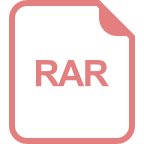
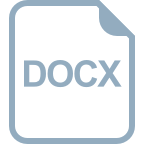
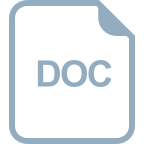











