ubantu中使用QT按键引用DLL文件中函数
时间: 2024-05-12 16:14:01 浏览: 226
在Ubuntu中使用QT调用DLL文件中的函数需要使用Qt的插件框架。Qt提供了一个名为QPluginLoader的类,可以动态加载共享库并获取其导出的符号。下面是一个简单的示例代码:
```cpp
#include <QApplication>
#include <QPluginLoader>
#include "plugininterface.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 加载插件
QPluginLoader pluginLoader("/path/to/plugin.so");
if (!pluginLoader.load()) {
qDebug() << "Failed to load plugin:" << pluginLoader.errorString();
return -1;
}
// 获取插件接口
PluginInterface* plugin = qobject_cast<PluginInterface*>(pluginLoader.instance());
if (!plugin) {
qDebug() << "Failed to get plugin interface";
return -1;
}
// 调用插件的函数
plugin->doSomething();
return a.exec();
}
```
其中,`PluginInterface`是一个纯虚基类,定义了插件的接口:
```cpp
class PluginInterface
{
public:
virtual ~PluginInterface() {}
virtual void doSomething() = 0;
};
```
在插件中,需要实现这个接口,并导出一个名为`createPlugin`的函数,用于创建插件的实例:
```cpp
#include "plugininterface.h"
class MyPlugin : public PluginInterface
{
public:
virtual void doSomething() override {
// do something
}
};
extern "C" PluginInterface* createPlugin()
{
return new MyPlugin();
}
```
最后,使用以下命令编译插件并链接到QT程序中:
```
g++ -shared -fPIC -o plugin.so plugin.cpp -I/path/to/qt/include -L/path/to/qt/lib -lQt5Core
```
其中,`/path/to/qt`是QT的安装路径。
阅读全文
相关推荐


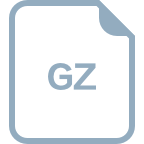













