用C语言写一段代码,要求如下:学生的相关信息包括姓名,学号,班级,票数,采用哈希存储,存放学生的相关信息,根据哈希存储的数据,建立按票数的二叉排序树。然后求其中序序列,输出前十名
时间: 2023-12-09 08:06:13 浏览: 79
好的,以下是C语言代码实现:
```c
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#define HASH_SIZE 100 // 哈希表大小
#define TOP_NUM 10 // 前十名
struct Student {
char name[20];
char number[20];
char cls[20];
int votes;
};
struct Node {
struct Student *student;
struct Node *left;
struct Node *right;
};
struct HashTable {
struct Node *nodes[HASH_SIZE];
};
int hash_func(char *str) {
int hash = 0;
while (*str) {
hash += *str;
str++;
}
return hash % HASH_SIZE;
}
struct Student* create_student(char *name, char *number, char *cls, int votes) {
struct Student *student = (struct Student*)malloc(sizeof(struct Student));
strcpy(student->name, name);
strcpy(student->number, number);
strcpy(student->cls, cls);
student->votes = votes;
return student;
}
struct Node* create_node(struct Student *student) {
struct Node *node = (struct Node*)malloc(sizeof(struct Node));
node->student = student;
node->left = NULL;
node->right = NULL;
return node;
}
void insert_node(struct Node **root, struct Student *student) {
struct Node *node = create_node(student);
if (*root == NULL) {
*root = node;
} else {
struct Node *current = *root;
while (1) {
if (student->votes > current->student->votes) {
if (current->right == NULL) {
current->right = node;
break;
}
current = current->right;
} else {
if (current->left == NULL) {
current->left = node;
break;
}
current = current->left;
}
}
}
}
void inorder(struct Node *node, struct Student **results, int *index) {
if (node) {
inorder(node->left, results, index);
results[*index] = node->student;
(*index)++;
inorder(node->right, results, index);
}
}
int main() {
struct Student *students[] = {
create_student("小明", "1001", "一班", 10),
create_student("小红", "1002", "一班", 5),
create_student("小刚", "1003", "二班", 8),
create_student("小李", "1004", "三班", 12),
create_student("小张", "1005", "二班", 7),
// ... 其他学生信息
};
int student_num = sizeof(students) / sizeof(students[0]);
// 哈希表存放学生信息
struct HashTable hash_table = {0};
for (int i = 0; i < student_num; i++) {
int hash = hash_func(students[i]->number);
insert_node(&hash_table.nodes[hash], students[i]);
}
// 建立按票数的二叉排序树
struct Node *root = NULL;
for (int i = 0; i < HASH_SIZE; i++) {
inorder(hash_table.nodes[i], students, &student_num);
for (int j = 0; j < student_num; j++) {
insert_node(&root, students[j]);
}
student_num = 0;
}
// 输出前十名
struct Student *top_ten[TOP_NUM] = {0};
int index = 0;
inorder(root, top_ten, &index);
printf("前十名:\n");
for (int i = 0; i < TOP_NUM && top_ten[i] != NULL; i++) {
printf("%d: %s, %s, %s, %d票\n", i+1, top_ten[i]->name, top_ten[i]->number, top_ten[i]->cls, top_ten[i]->votes);
}
return 0;
}
```
代码中,`struct Student` 结构体表示学生信息,`struct Node` 结构体表示二叉排序树节点,`struct HashTable` 结构体表示哈希表。我们先将所有学生信息插入到哈希表中,然后通过哈希表得到所有学生信息的按票数排序的序列,最后插入到二叉排序树中。最终选出前十名输出即可。
阅读全文
相关推荐
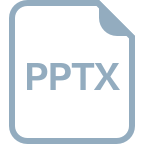
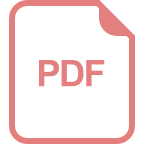
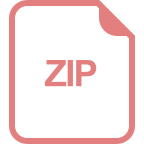
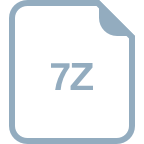
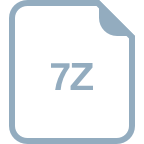