学生成绩管理系统c语言文件链表
时间: 2023-07-27 10:23:44 浏览: 129
好的,我可以为你提供一些关于学生成绩管理系统的C语言文件链表的基本思路。
首先,我们需要定义一个学生结构体,包含学生的基本信息和成绩信息。
```c
typedef struct student {
int id; // 学号
char name[20]; // 姓名
float score; // 成绩
struct student *next; // 下一个学生的指针
} Student;
```
然后,我们需要定义一个链表,用于存储所有的学生信息。
```c
Student *head = NULL; // 链表头指针
Student *tail = NULL; // 链表尾指针
```
接下来,我们可以通过读取文件的方式将学生信息读入到链表中。具体来说,我们可以定义一个函数,用于读取文件中的学生信息,并将其添加到链表中。
```c
void load_students(char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error opening file %s\n", filename);
return;
}
while (!feof(fp)) {
Student *new_student = (Student *) malloc(sizeof(Student));
fscanf(fp, "%d %s %f", &new_student->id, new_student->name, &new_student->score);
new_student->next = NULL;
if (head == NULL) {
head = new_student;
tail = new_student;
} else {
tail->next = new_student;
tail = new_student;
}
}
fclose(fp);
}
```
这个函数会打开指定的文件,逐行读取文件中的学生信息,并将其添加到链表的末尾。需要注意的是,我们需要为每个新的学生动态分配内存。
最后,我们还可以定义一些其他的函数,比如添加学生信息、删除学生信息、修改学生信息和查询学生信息等等。
```c
// 添加学生信息
void add_student(Student *new_student) {
new_student->next = NULL;
if (head == NULL) {
head = new_student;
tail = new_student;
} else {
tail->next = new_student;
tail = new_student;
}
}
// 删除学生信息
void delete_student(int id) {
if (head == NULL) {
return;
}
Student *curr = head;
Student *prev = NULL;
while (curr != NULL) {
if (curr->id == id) {
if (prev == NULL) {
head = curr->next;
} else {
prev->next = curr->next;
}
if (curr == tail) {
tail = prev;
}
free(curr);
return;
}
prev = curr;
curr = curr->next;
}
}
// 修改学生信息
void modify_student(int id, char *name, float score) {
Student *curr = head;
while (curr != NULL) {
if (curr->id == id) {
strcpy(curr->name, name);
curr->score = score;
return;
}
curr = curr->next;
}
}
// 查询学生信息
void search_student(int id) {
Student *curr = head;
while (curr != NULL) {
if (curr->id == id) {
printf("ID: %d\nName: %s\nScore: %.2f\n", curr->id, curr->name, curr->score);
return;
}
curr = curr->next;
}
printf("Student with ID %d not found.\n", id);
}
```
这些函数可以通过调用来实现学生成绩管理系统的基本功能。需要注意的是,我们需要在程序结束时,将链表中的学生信息写入到文件中,以保证数据的持久化。
希望这些代码可以对你有所帮助!
阅读全文
相关推荐
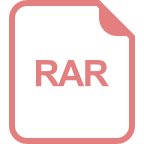
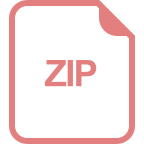
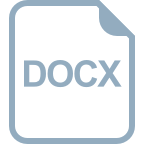















