golang如何集成Terraform
时间: 2023-07-26 13:15:10 浏览: 269
要在golang中集成Terraform,你可以使用Terraform的Go SDK - Terraform Plugin SDK。该SDK允许你在golang中编写自定义插件,以与Terraform进行交互。
以下是基本的步骤:
1. 安装Terraform
2. 创建一个新的golang项目
3. 导入Terraform Plugin SDK
4. 实现Terraform插件接口
5. 构建和安装插件
6. 在Terraform配置中使用插件
这里提供一个简单的示例,你可以参考此示例来开始你的项目:
```go
package main
import (
"context"
"fmt"
"os"
"github.com/hashicorp/terraform-plugin-sdk/helper/schema"
"github.com/hashicorp/terraform-plugin-sdk/plugin"
"github.com/hashicorp/terraform-plugin-sdk/terraform"
)
func main() {
plugin.Serve(&plugin.ServeOpts{
ProviderFunc: func() terraform.ResourceProvider {
return &schema.Provider{
ResourcesMap: map[string]*schema.Resource{
"myresource": &schema.Resource{
Create: resourceCreate,
Read: resourceRead,
Update: resourceUpdate,
Delete: resourceDelete,
Schema: map[string]*schema.Schema{
"name": &schema.Schema{
Type: schema.TypeString,
Description: "The name of the resource.",
Required: true,
},
},
},
},
}
},
})
}
func resourceCreate(ctx context.Context, d *schema.ResourceData, meta interface{}) diag.Diagnostics {
name := d.Get("name").(string)
if name == "" {
return diag.Errorf("name must be set")
}
d.SetId(name)
return nil
}
func resourceRead(ctx context.Context, d *schema.ResourceData, meta interface{}) diag.Diagnostics {
return nil
}
func resourceUpdate(ctx context.Context, d *schema.ResourceData, meta interface{}) diag.Diagnostics {
return nil
}
func resourceDelete(ctx context.Context, d *schema.ResourceData, meta interface{}) diag.Diagnostics {
return nil
}
```
这个示例中创建了一个自定义资源 `myresource` ,并实现了它的 `create`、`read`、`update`、`delete` 四个方法。你可以根据你的需求来编写你自己的插件。
要构建和安装插件,你可以使用以下命令:
```shell
go build -o ~/.terraform.d/plugins/example.com/mycompany/myprovider/1.0/darwin_amd64/terraform-provider-myprovider
```
在Terraform配置中使用插件,你需要指定插件的名称和版本:
```terraform
terraform {
required_providers {
myprovider = {
source = "example.com/mycompany/myprovider"
version = "1.0"
}
}
}
resource "myprovider_myresource" "example" {
name = "example"
}
```
这就是一个简单的集成Terraform的golang项目。你可以通过这个示例来进一步了解如何开发你自己的插件。
阅读全文
相关推荐
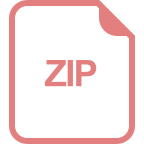
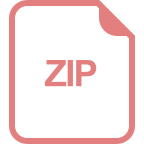
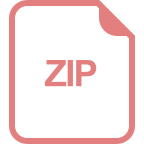
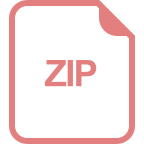
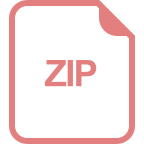
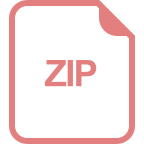
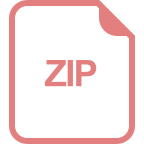
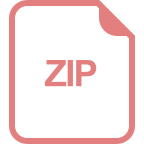
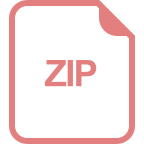
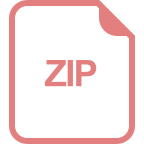
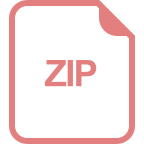
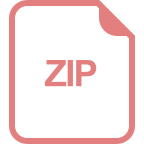
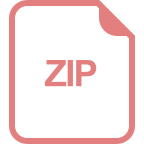
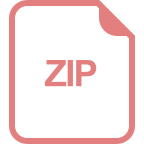
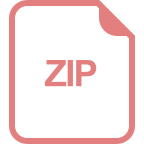
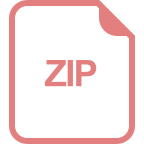
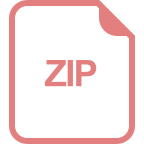
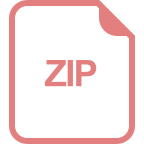