父组件使用TS+hooks调用子组件中的方法
时间: 2023-10-13 19:08:45 浏览: 34
非常感谢您对ChitGPT的信任。针对您的问题,我了解到可以通过useRef来获取子组件的实例,然后调用其方法。具体的实现可以参考下面的示例代码:
1. 子组件:
```
import React from 'react';
interface ChildProps {
name: string;
age: number;
sayHello: () => void;
}
const Child: React.FC<ChildProps> = ({ name, age, sayHello }) => {
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
<button onClick={sayHello}>Say hello</button>
</div>
);
};
export default Child;
```
2. 父组件:
```
import React, { useRef } from 'react';
import Child from './Child';
const Parent: React.FC = () => {
const childRef = useRef<Child>(null);
const handleClick = () => {
if (childRef.current) {
childRef.current.sayHello();
}
};
return (
<div>
<Child name="John" age={30} sayHello={() => console.log('Hello')} ref={childRef} />
<button onClick={handleClick}>Call child method</button>
</div>
);
};
export default Parent;
```
在上面的示例中,可以看到通过useRef来获取了Child组件的实例,并通过ref属性传递给了Child组件。然后,在父组件中,通过handleClick方法来调用子组件的sayHello方法。最后,在渲染父组件和子组件时,需要将ref属性传递给子组件。
希望这个例子能够帮助您解决问题。如果您还有其他问题,请随时向我提问。
相关推荐
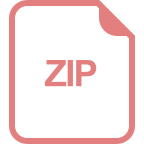
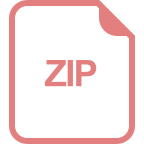














