python 中如何去除列表中的1。
时间: 2024-05-01 14:21:25 浏览: 19
可以使用列表推导式来去除列表中的1,示例如下:
```python
my_list = [1, 2, 3, 1, 4, 1]
new_list = [x for x in my_list if x != 1]
print(new_list) # 输出 [2, 3, 4]
```
在这个示例中,使用了列表推导式来创建一个新的列表 `new_list`,其中只包含原列表 `my_list` 中不等于1的元素。
相关问题
如何在Python中去除列表中的重复元素?
以下是Python中去除列表中重复元素的几种方法:
1.使用set()函数去重,再转换回列表类型
```python
lst = [1, 2, 3, 2, 4, 1]
new_lst = list(set(lst))
print(new_lst) # 输出:[1, 2, 3, 4]
```
2.使用列表推导式去重
```python
lst = [1, 2, 3, 2, 4, 1]
new_lst = []
[new_lst.append(i) for i in lst if i not in new_lst]
print(new_lst) # 输出:[1, 2, 3, 4]
```
3.使用字典去重
```python
lst = [1, 2, 3, 2, 4, 1]
new_lst = list(dict.fromkeys(lst))
print(new_lst) # 输出:[1, 2, 3, 4]
```
4.使用collections模块中的OrderedDict去重
```python
from collections import OrderedDict
lst = [1, 2, 3, 2, 4, 1]
new_lst = list(OrderedDict.fromkeys(lst))
print(new_lst) # 输出:[1, 2, 3, 4]
```
python怎么去掉列表中的中括号
要去掉一个列表中的中括号,可以使用字符串格式化或者join方法将其转换为字符串,例如:
```python
my_list = ['apple', 'banana', 'orange']
print(str(my_list).strip('[]')) # 输出:'apple', 'banana', 'orange'
print(', '.join(my_list)) # 输出:'apple, banana, orange'
```
在第一种方法中,我们首先使用str()将列表转换为字符串,然后使用strip()方法去掉字符串两端的中括号。在第二种方法中,我们使用join()方法将列表中的元素连接成一个字符串,并且使用逗号和空格作为分隔符。
阅读全文
相关推荐
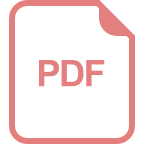
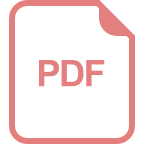













