java开启线程的方法有几种案例
时间: 2023-08-01 11:06:09 浏览: 113
Java中开启线程的方法有以下几种:
1. 继承Thread类并重写run方法:
```java
public class MyThread extends Thread {
public void run() {
// 线程运行的代码
}
}
// 创建并启动线程的方式
MyThread thread = new MyThread();
thread.start();
```
2. 实现Runnable接口并重写run方法:
```java
public class MyRunnable implements Runnable {
public void run() {
// 线程运行的代码
}
}
// 创建并启动线程的方式
MyRunnable runnable = new MyRunnable();
Thread thread = new Thread(runnable);
thread.start();
```
3. 实现Callable接口并重写call方法:
```java
public class MyCallable implements Callable<Integer> {
public Integer call() throws Exception {
// 线程运行的代码
return 1;
}
}
// 创建并启动线程的方式
MyCallable callable = new MyCallable();
ExecutorService executor = Executors.newSingleThreadExecutor();
Future<Integer> future = executor.submit(callable);
```
4. 使用匿名内部类:
```java
Thread thread = new Thread(new Runnable() {
public void run() {
// 线程运行的代码
}
});
thread.start();
```
5. 使用Lambda表达式:
```java
Thread thread = new Thread(() -> {
// 线程运行的代码
});
thread.start();
```
需要注意的是,以上方法中前三种都可以实现线程的基本功能。而后两种方法是比较简洁的写法,适用于需要开启较为简单的线程使用。
阅读全文
相关推荐
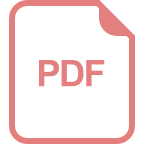
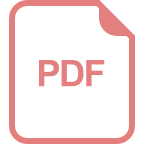
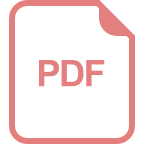
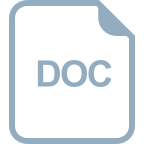
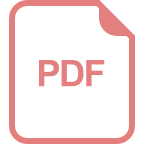
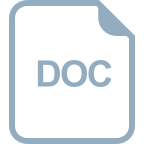
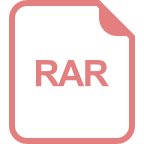
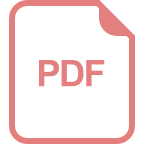
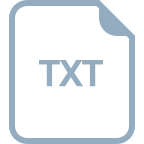
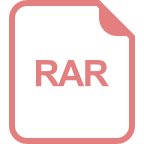
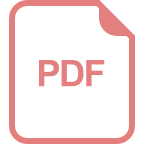
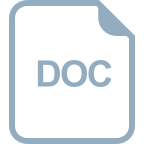
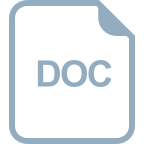
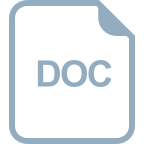
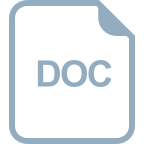
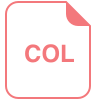
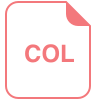