使用Spring Security和MySQL进行身份验证
时间: 2023-07-25 15:43:50 浏览: 211
要使用Spring Security和MySQL进行身份验证,您需要完成以下步骤:
1. 添加Spring Security和MySQL的依赖。您可以在Maven或Gradle中添加以下依赖:
```xml
<!-- Spring Security -->
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>5.5.1</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>5.5.1</version>
</dependency>
<!-- MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
```
2. 创建一个用户表。您需要在MySQL中创建一个用户表,用于存储用户信息和密码。例如:
```sql
CREATE TABLE users (
id INT NOT NULL AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
password VARCHAR(100) NOT NULL,
enabled TINYINT NOT NULL,
PRIMARY KEY (id),
UNIQUE KEY unique_username (username)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
3. 实现UserDetailsService接口。您需要创建一个实现UserDetailsService接口的类,用于查询用户信息并返回UserDetails对象。例如:
```java
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
List<GrantedAuthority> authorities = new ArrayList<>();
for (Role role : user.getRoles()) {
authorities.add(new SimpleGrantedAuthority(role.getName()));
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), user.isEnabled(), true, true, true, authorities);
}
}
```
其中,UserRepository是一个自定义的JpaRepository,用于从数据库中查询用户信息。User类包含了用户名、密码、是否可用等信息,以及一个Role列表,用于存储此用户所拥有的角色。
4. 配置Spring Security。您需要在Spring Security的配置文件中指定UserDetailsService和PasswordEncoder,并配置身份验证规则。例如:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("USER", "ADMIN")
.antMatchers("/", "/login").permitAll()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/")
.and()
.logout()
.logoutUrl("/logout")
.logoutSuccessUrl("/")
.and()
.csrf().disable();
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
在上述配置中,我们使用了BCryptPasswordEncoder作为PasswordEncoder的实现类,用于对用户密码进行加密。在configure(HttpSecurity http)方法中,我们设置了不同URL需要的角色权限,并指定了登录页、登出URL等相关信息。
5. 创建登录页和主页。最后,您需要创建一个登录页和主页,用于用户登录和展示相关信息。例如:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form method="post" action="/login">
<div>
<label>Username:</label>
<input type="text" name="username">
</div>
<div>
<label>Password:</label>
<input type="password" name="password">
</div>
<button type="submit">Login</button>
</form>
</body>
</html>
```
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Home</title>
</head>
<body>
<h1>Welcome!</h1>
<p>Logged in as: <strong><sec:authentication property="name"/></strong></p>
<p>Roles: <strong><sec:authentication property="authorities"/></strong></p>
<a href="/logout">Logout</a>
</body>
</html>
```
以上就是使用Spring Security和MySQL进行身份验证的基本步骤。您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
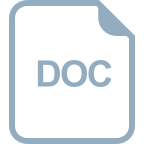
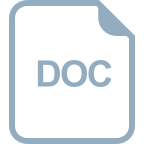
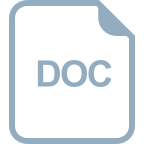
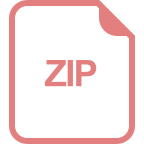
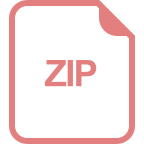
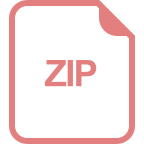
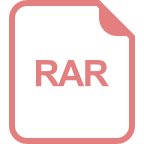
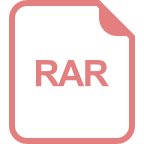
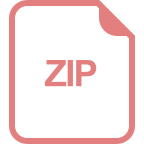
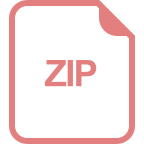
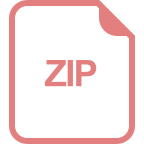
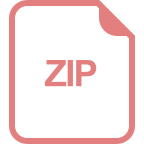
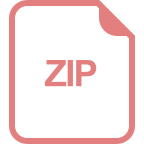
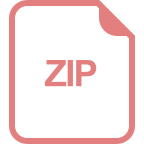
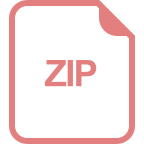
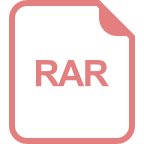

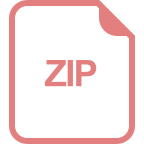
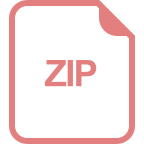