基于标准库定时中断产生一个周期为两秒的呼吸灯
时间: 2024-12-06 20:20:47 浏览: 6
基于标准库实现一个周期为两秒的呼吸灯通常涉及到操作系统提供的计时功能,比如Linux下的`sleep`函数或者C++11引入的`std::this_thread::sleep_for`。这里我会提供一个基本的伪代码示例来说明如何在C++中通过循环实现这个效果:
```cpp
#include <iostream>
#include <thread> // 使用C++11的线程库
#include <chrono> // 包含时间相关的头文件
// 定义呼吸灯状态
enum class LightState { OFF, ON, FLICKER };
void blink_light(LightState state) {
while (true) {
switch (state) {
case LightState::OFF:
std::cout << "Light is off.\n";
break;
case LightState::ON:
std::cout << "Light is on.\n";
// 模拟LED亮起
// 这里通常是控制硬件操作,例如GPIO设置等
// 对于软件模拟,可以简单打印字符代替
sleep_for(std::chrono::seconds(1));
std::cout << "\rLight blinked once.\n";
break;
case LightState::FLICKER:
for (int i = 0; i <= 2; ++i) {
if (i % 2 == 0) {
std::cout << "Light is slowly blinking.\n";
sleep_for(std::chrono::seconds(0.5)); // 慢慢闪烁
} else {
std::cout << "Light is off.\n";
sleep_for(std::chrono::seconds(1)); // 灯灭间隔
}
}
break;
}
// 更新状态,例如每两秒切换一次
std::this_thread::sleep_for(std::chrono::seconds(2));
state = (state + 1) % 3; // 循环状态:OFF -> ON -> FLICKER
}
}
int main() {
LightState initial_state = LightState::OFF;
std::thread breathing_thread(blink_light, initial_state);
// 主线程可以做其他事情...
while (breathing_thread.joinable()) {}
return 0;
}
```
阅读全文
相关推荐
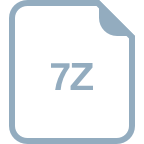
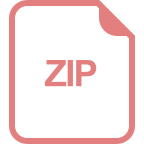
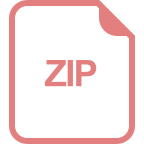
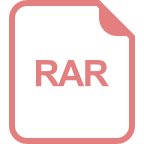
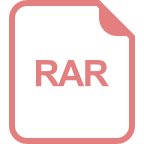
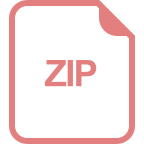
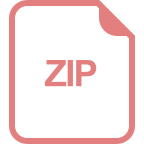
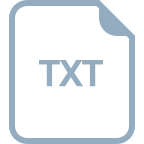
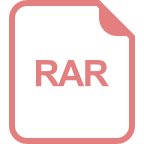
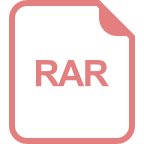
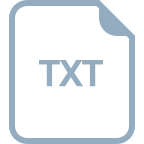
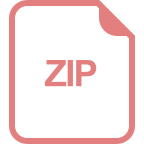