uniapp真机 文件流blob下载
时间: 2024-08-02 12:01:37 浏览: 257
在uniApp中,文件流Blob(二进制大对象)下载通常发生在需要将服务器返回的数据(比如图片、视频等)保存到本地或者提供给用户直接下载的情况。以下是一个简单的示例步骤:
1. 获取服务器响应:首先从服务器获取一个包含文件数据的Blob对象。这可以是通过网络请求API得到的结果。
```javascript
let blob = await yourAPI.downloadFile();
```
2. 创建下载链接:创建一个隐藏的`<a>`标签,并设置其href属性为Blob URL,然后触发点击事件来开始下载。
```javascript
let link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = 'yourFileName'; // 下载名称
link.style.display = 'none';
document.body.appendChild(link);
link.click();
```
3. 清理资源:当下载完成后,记得清除`window.URL.createObjectURL`生成的临时URL,释放内存。
```javascript
window.URL.revokeObjectURL(link.href);
document.body.removeChild(link);
```
相关问题
uniapp下载文件为blob怎么处理
在uniapp中,可以使用uni.downloadFile()方法来下载文件,下载完成后会返回一个临时文件路径,可以使用uni.getFileSystemManager()方法将其转成blob对象。
以下是实现代码:
```
uni.downloadFile({
url: 'your download url',
success: (res) => {
if (res.statusCode === 200) {
uni.getFileSystemManager().readFile({
filePath: res.tempFilePath,
encoding: 'base64',
success: (data) => {
let base64 = 'data:image/jpeg;base64,' + data.data
let blob = this.base64ToBlob(base64)
console.log(blob)
}
})
}
}
})
// base64转blob
base64ToBlob(base64) {
let arr = base64.split(',')
let type = arr[0].match(/:(.*?);/)[1]
let bstr = atob(arr[1])
let n = bstr.length
let u8arr = new Uint8Array(n)
while (n--) {
u8arr[n] = bstr.charCodeAt(n)
}
return new Blob([u8arr], { type: type })
}
```
这样就可以将下载下来的文件转成blob对象了。
uniapp下载文件为blob怎么打开
在uniapp中,可以使用uni.previewImage()方法来预览图片,可以使用uni.openDocument()方法来打开其他类型的文件。
以下是实现代码:
```
uni.downloadFile({
url: 'your download url',
success: (res) => {
if (res.statusCode === 200) {
uni.getFileSystemManager().readFile({
filePath: res.tempFilePath,
encoding: 'base64',
success: (data) => {
let base64 = 'data:image/jpeg;base64,' + data.data
let blob = this.base64ToBlob(base64)
console.log(blob)
if (blob.type.indexOf('image') !== -1) {
uni.previewImage({
urls: [base64]
})
} else {
uni.openDocument({
filePath: res.tempFilePath
})
}
}
})
}
}
})
// base64转blob
base64ToBlob(base64) {
let arr = base64.split(',')
let type = arr[0].match(/:(.*?);/)[1]
let bstr = atob(arr[1])
let n = bstr.length
let u8arr = new Uint8Array(n)
while (n--) {
u8arr[n] = bstr.charCodeAt(n)
}
return new Blob([u8arr], { type: type })
}
```
这样就可以根据文件类型来选择预览图片或打开其他类型的文件了。
阅读全文
相关推荐
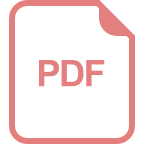
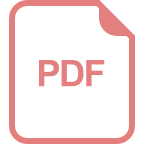













