用栈逆置一个单链表。利用一个栈将一个单链表(al,a2,...,an)逆置为(an, an-1,...al)。用c语言实现
时间: 2024-10-18 07:14:26 浏览: 26
在C语言中,我们可以使用栈来逆置一个单链表。基本思路是遍历链表,依次将节点压入栈中,然后弹出栈顶元素作为新的头节点,并更新其后的指针指向当前弹出的节点。以下是具体的步骤和代码实现:
首先,我们需要定义链表节点结构和栈结构:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct Stack {
Node* top;
int size;
} Stack;
```
接着,我们创建两个辅助函数,一个用于初始化栈,另一个用于将节点压入栈:
```c
void push(Stack* stack, Node* node) {
if (stack->top == NULL) {
stack->top = node;
} else {
node->next = stack->top;
stack->top = node;
}
stack->size++;
}
Node* pop(Stack* stack) {
if (stack->top == NULL) {
return NULL;
}
Node* temp = stack->top;
stack->top = stack->top->next;
stack->size--;
return temp;
}
```
现在,我们可以开始逆置链表的过程:
```c
void reverseList(Node** head) {
Node* prev = NULL; // 初始化前驱节点
Node* current = *head; // 初始化当前节点
Stack s; // 创建栈
while (current != NULL) {
push(&s, current); // 将节点压入栈
prev = current; // 更新前驱节点
current = current->next; // 移动当前节点
}
*head = pop(&s); // 从栈顶取出新头节点
current = prev; // 新的头节点之后的节点
while (current != NULL) {
current->next = pop(&s); // 取出下一个节点并连接上
current = current->next;
}
}
```
以上代码会把给定的链表`*head`逆置并返回逆置后的链表首节点。记得在调用这个函数之前,需要先设置好链表的头节点。
阅读全文
相关推荐
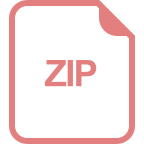
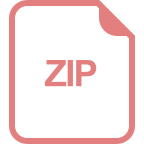
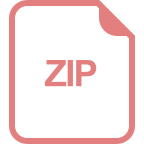



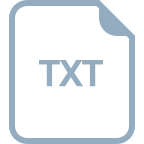
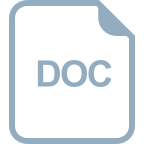










