用vue3.0写一个导航网页
时间: 2023-10-01 16:03:41 浏览: 40
好的,下面是一个简单的导航网页示例,使用Vue3.0和Tailwind CSS框架。
首先,我们需要创建一个Vue实例,并在HTML中引入Vue和Tailwind CSS:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>导航网页</title>
<!-- 引入Vue和Tailwind CSS -->
<script src="https://unpkg.com/vue@next"></script>
<link href="https://cdn.jsdelivr.net/npm/tailwindcss/dist/tailwind.min.css" rel="stylesheet">
</head>
<body>
<!-- Vue应用 -->
<div id="app">
<!-- 导航栏 -->
<nav class="bg-gray-800">
<div class="max-w-7xl mx-auto px-2 sm:px-6 lg:px-8">
<div class="relative flex items-center justify-between h-16">
<div class="absolute inset-y-0 left-0 flex items-center sm:hidden">
<!-- 移动端菜单按钮 -->
<button @click="showMenu = !showMenu" type="button" class="inline-flex items-center justify-center p-2 rounded-md text-gray-400 hover:text-white hover:bg-gray-700 focus:outline-none focus:ring-2 focus:ring-inset focus:ring-white" aria-controls="mobile-menu" aria-expanded="false">
<span class="sr-only">Open main menu</span>
<!-- 菜单图标 -->
<svg :class="{'hidden': showMenu, 'block': !showMenu}" class="block h-6 w-6" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke="currentColor" aria-hidden="true">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M4 6h16M4 12h16M4 18h16"/>
</svg>
<!-- 关闭图标 -->
<svg :class="{'hidden': !showMenu, 'block': showMenu}" class="block h-6 w-6" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke="currentColor" aria-hidden="true">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M6 18L18 6M6 6l12 12"/>
</svg>
</button>
</div>
<div class="flex-1 flex items-center justify-center sm:items-stretch sm:justify-start">
<div class="flex-shrink-0">
<!-- 网站Logo -->
<img class="block lg:hidden h-8 w-auto" src="https://tailwindui.com/img/logos/workflow-mark-indigo-500.svg" alt="Workflow">
<img class="hidden lg:block h-8 w-auto" src="https://tailwindui.com/img/logos/workflow-logo-indigo-500-mark-white-text.svg" alt="Workflow">
</div>
<div class="hidden sm:block sm:ml-6">
<div class="flex space-x-4">
<!-- 导航链接 -->
<a href="#" class="bg-gray-900 text-white px-3 py-2 rounded-md text-sm font-medium">首页</a>
<a href="#" class="text-gray-300 hover:bg-gray-700 hover:text-white px-3 py-2 rounded-md text-sm font-medium">博客</a>
<a href="#" class="text-gray-300 hover:bg-gray-700 hover:text-white px-3 py-2 rounded-md text-sm font-medium">关于</a>
<a href="#" class="text-gray-300 hover:bg-gray-700 hover:text-white px-3 py-2 rounded-md text-sm font-medium">联系我们</a>
</div>
</div>
</div>
</div>
</div>
<!-- 移动端菜单 -->
<div :class="{'block': showMenu, 'hidden': !showMenu}" class="sm:hidden" id="mobile-menu">
<div class="px-2 pt-2 pb-3 space-y-1">
<a href="#" class="bg-gray-900 text-white block px-3 py-2 rounded-md text-base font-medium">首页</a>
<a href="#" class="text-gray-300 hover:bg-gray-700 hover:text-white block px-3 py-2 rounded-md text-base font-medium">博客</a>
<a href="#" class="text-gray-300 hover:bg-gray-700 hover:text-white block px-3 py-2 rounded-md text-base font-medium">关于</a>
<a href="#" class="text-gray-300 hover:bg-gray-700 hover:text-white block px-3 py-2 rounded-md text-base font-medium">联系我们</a>
</div>
</div>
</nav>
<!-- 内容区域 -->
<main>
<div class="max-w-7xl mx-auto py-6 sm:px-6 lg:px-8">
<div class="px-4 py-6 sm:px-0">
<!-- 内容 -->
<h1 class="text-3xl font-bold mb-6">欢迎访问我的导航网页</h1>
<p class="text-lg mb-4">这里提供了一些有用的链接和资源,希望能对您有所帮助。</p>
<ul class="list-disc list-inside">
<li><a href="#">链接1</a></li>
<li><a href="#">链接2</a></li>
<li><a href="#">链接3</a></li>
<li><a href="#">链接4</a></li>
</ul>
</div>
</div>
</main>
</div>
<!-- Vue应用脚本 -->
<script>
const app = Vue.createApp({
data() {
return {
showMenu: false // 控制移动端菜单的显示和隐藏
}
}
}).mount('#app')
</script>
</body>
</html>
```
这个示例中包含了一个导航栏和一个内容区域。导航栏包含了一个移动端菜单按钮和一些导航链接,移动端菜单使用了Vue的动态绑定来控制显示和隐藏。内容区域包含了一些文本和链接。
你可以根据自己的需求修改示例中的内容和样式,达到你想要的效果。
相关推荐
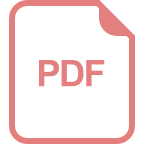
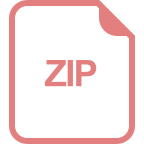














