input = getGrayPix_R(bit_image, false); try { ByteBuffer buffer = loadModelFile(this.getAssets(),MODEL_PATH); tflite = new Interpreter(buffer); tflite.run(input,output); String out_prnt = "output: "; for (int m=0;m<10;++m){ out_prnt += Float.toString(output[0][m]) + "\t"; } resut_f.setText(out_prnt); }catch (IOException ex){ Log.e(TAG, "Error loading TF Lite model.\n", ex); }
时间: 2024-02-15 21:28:23 浏览: 26
这段代码是将加载的图像输入到 TensorFlow Lite 模型中进行分类,并将输出结果显示在应用程序界面上。首先,调用 `getGrayPix_R` 函数将读取的图像转换为一个大小为 (1, 28, 28) 的三维浮点型数组 `input`,其中将 `isReverse` 参数设置为 false。然后,使用 `loadModelFile` 函数从 assets 文件夹中加载 TensorFlow Lite 模型,并创建一个 `Interpreter` 对象 `tflite`。接着,调用 `tflite.run` 函数将输入数据 `input` 传递给模型进行分类,并将分类结果存储在 `output` 数组中。接下来,使用一个循环遍历 `output` 数组中的每个元素,并将其转换为字符串类型,存储在 `out_prnt` 变量中。最后,使用 `setText` 函数将 `out_prnt` 显示在应用程序界面上的 `TextView` 中,以便用户查看分类结果。如果在加载 TensorFlow Lite 模型文件时发生错误,则使用 `Log.e` 函数输出错误信息。
相关问题
ByteBuffer buffer = ByteBuffer.wrap(buff); buffer.order(ByteOrder.BIG_ENDIAN); 如何重新从头读取
要重新从头开始读取 ByteBuffer,可以使用 `buffer.rewind()` 方法将缓冲区的位置重置为0。这样可以让后续的读取操作重新从缓冲区的开头开始。以下是一个示例代码:
```java
ByteBuffer buffer = ByteBuffer.wrap(buff);
buffer.order(ByteOrder.BIG_ENDIAN);
// 重新从头开始读取
buffer.rewind();
// 进行读取操作
// ...
```
在调用 `buffer.rewind()` 方法后,你可以按照需要进行后续的读取操作。缓冲区的位置将会被重置为0,这样下一次的读取操作将会从缓冲区的开头开始。
package com.example.myapplication; import androidx.appcompat.app.AppCompatActivity; import android.content.res.AssetFileDescriptor; import android.content.res.AssetManager; import android.os.Bundle; import android.util.Log; import android.widget.TextView; import org.tensorflow.lite.Interpreter; import java.io.FileInputStream; import java.io.IOException; import java.nio.ByteBuffer; import java.nio.MappedByteBuffer; import java.nio.channels.FileChannel; public class logist extends AppCompatActivity { private static final String MODEL_PATH = "model_logist.tflite"; private static final String TAG = "Interpreter"; private Interpreter tflite; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_logist); TextView resut_f = findViewById(R.id.resut_f); float output[][] = new float[1][1]; float[][] input = {{(float) 0.4977424,(float) 0.3815156 , (float) 0.92981267 ,(float) 0.30464694, (float) 0.0306613, (float) 0.2767251, (float) 0.42961425, (float) 0.10500819 ,(float) 0.6788244 , (float) 0.80088454 ,(float) 0.744523 , (float) 0.8165212, (float) 0.91727537, (float) 0.5188435 , (float) 0.0448584}}; // input[0] = [9]; // try { ByteBuffer buffer = loadModelFile(this.getAssets(), MODEL_PATH); tflite = new Interpreter(buffer); tflite.run(input, output); resut_f.setText(Float.toString(output[0][0])); } catch (IOException ex) { Log.e(TAG, "Error loading TF Lite model.\n", ex); } } /** 从assets目录加载TF Lite模型. */ private static MappedByteBuffer loadModelFile(AssetManager assetManager, String modelPath) throws IOException { try (AssetFileDescriptor fileDescriptor = assetManager.openFd(modelPath); FileInputStream inputStream = new FileInputStream(fileDescriptor.getFileDescriptor())) { FileChannel fileChannel = inputStream.getChannel(); long startOffset = fileDescriptor.getStartOffset(); long declaredLength = fileDescriptor.getDeclaredLength(); return fileChannel.map(FileChannel.MapMode.READ_ONLY, startOffset, declaredLength); } } }
这是一个使用 TensorFlow Lite 框架加载机器学习模型并进行推理的 Android 应用程序。具体来说,它加载了一个名为 "model_logist.tflite" 的机器学习模型,该模型可以接受一个包含 15 个浮点数的输入数组,并返回一个包含一个浮点数的输出数组。输入和输出的值分别通过变量 input 和 output 进行存储,并且在应用程序的界面上显示了该输出值。
相关推荐
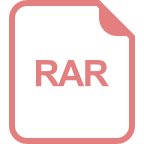
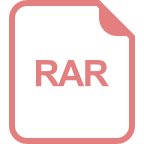
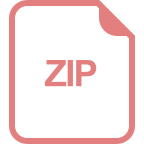













