c语言生产者消费者问题
时间: 2023-08-24 12:28:46 浏览: 100
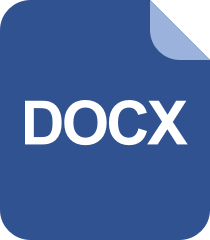
利用数组解决生产者消费者问题
C语言生产者消费者问题是一个经典的多线程同步问题,其场景模拟了生产者和消费者在共享有限缓冲区时的操作。具体来说,生产者向缓冲区生产产品,消费者从缓冲区消费产品,两者需要协调合作,保证生产和消费的平衡,避免缓冲区溢出或者消费者阻塞等问题。
解决该问题的方法有很多种,其中最常用的是使用互斥锁和条件变量。生产者和消费者共享一个互斥锁,用于保证缓冲区的互斥访问。同时,使用两个条件变量,分别表示缓冲区非空和非满。当缓冲区非空时,消费者可以从缓冲区中取出产品;当缓冲区非满时,生产者可以将产品放入缓冲区中。通过这种方式,生产者和消费者可以协调合作,避免了缓冲区溢出或者消费者阻塞等问题。
以下是一个简单的C语言生产者消费者问题的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = 0, out = 0;
int count = 0;
pthread_mutex_t mutex;
pthread_cond_t not_full;
pthread_cond_t not_empty;
void *producer(void *arg)
{
int item;
while (1) {
item = rand() % 1000;
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) {
pthread_cond_wait(¬_full, &mutex);
}
buffer[in] = item;
in = (in + 1) % BUFFER_SIZE;
count++;
printf("Producer produces %d, count = %d\n", item, count);
pthread_cond_signal(¬_empty);
pthread_mutex_unlock(&mutex);
sleep(rand() % 3);
}
}
void *consumer(void *arg)
{
int item;
while (1) {
pthread_mutex_lock(&mutex);
while (count == 0) {
pthread_cond_wait(¬_empty, &mutex);
}
item = buffer[out];
out = (out + 1) % BUFFER_SIZE;
count--;
printf("Consumer consumes %d, count = %d\n", item, count);
pthread_cond_signal(¬_full);
pthread_mutex_unlock(&mutex);
sleep(rand() % 3);
}
}
int main()
{
pthread_t tid1, tid2;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(¬_full, NULL);
pthread_cond_init(¬_empty, NULL);
pthread_create(&tid1, NULL, producer, NULL);
pthread_create(&tid2, NULL, consumer, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(¬_full);
pthread_cond_destroy(¬_empty);
return 0;
}
```
在该代码中,我们使用了互斥锁和条件变量来协调生产者和消费者的操作。其中,互斥锁用于保证缓冲区的互斥访问,条件变量`not_full`表示缓冲区非满,条件变量`not_empty`表示缓冲区非空。在生产者和消费者的操作中,分别使用`pthread_cond_wait`和`pthread_cond_signal`来等待和唤醒条件变量,从而协调生产者和消费者的操作。
阅读全文
相关推荐
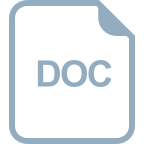
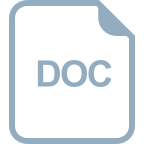
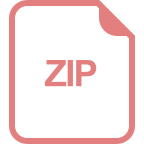


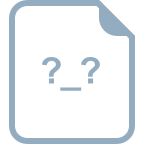
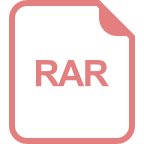
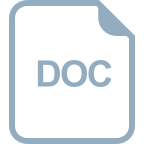
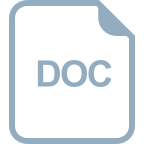





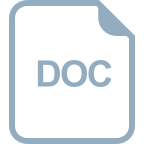
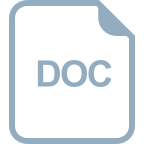
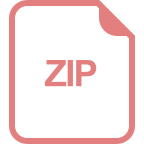