用python写一个五子棋代码
时间: 2023-05-22 22:05:21 浏览: 104
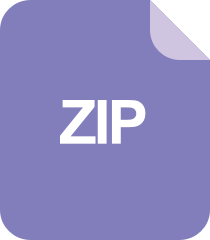
基于Python的五子棋程序代码.zip

好的,以下是一个五子棋代码的示例,使用Python实现:
```
# 定义一个棋盘类
class Board:
def __init__(self):
self.width = 15
self.height = 15
self.board = [[' ' for y in range(self.height)] for x in range(self.width)]
def print_board(self):
print(' 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15')
print(' +' + '---+'*15)
for y in range(self.height):
print(chr(y+65) + ' |', end='')
for x in range(self.width):
print(f' {self.board[x][y]} |', end='')
print()
print(' +' + '---+'*15)
def place(self, x, y, piece):
self.board[x][y] = piece
def get(self, x, y):
return self.board[x][y]
def is_valid_move(self, x, y):
return x >= 0 and x < self.width and y >= 0 and y < self.height and self.board[x][y] == ' '
# 定义一个游戏类
class Game:
def __init__(self):
self.board = Board()
self.players = ['X', 'O']
self.current_player = self.players[0]
def switch_player(self):
if self.current_player == self.players[0]:
self.current_player = self.players[1]
else:
self.current_player = self.players[0]
def play(self):
while True:
self.board.print_board()
move = input(f'{self.current_player}, enter your move (e.g. A1): ')
if len(move) != 2 or not move[0].isalpha() or not move[1].isdigit():
print('Invalid move, please try again.')
continue
x = ord(move[0].upper()) - 65
y = int(move[1]) - 1
if not self.board.is_valid_move(x, y):
print('Invalid move, please try again.')
continue
self.board.place(x, y, self.current_player)
if self.is_winner():
self.board.print_board()
print(f'{self.current_player} wins!')
break
self.switch_player()
def is_winner(self):
for y in range(self.board.height):
for x in range(self.board.width):
if x + 4 < self.board.width and \
self.board.get(x,y) == self.current_player and \
self.board.get(x+1,y) == self.current_player and \
self.board.get(x+2,y) == self.current_player and \
self.board.get(x+3,y) == self.current_player and \
self.board.get(x+4,y) == self.current_player:
return True
elif y + 4 < self.board.height and \
self.board.get(x,y) == self.current_player and \
self.board.get(x,y+1) == self.current_player and \
self.board.get(x,y+2) == self.current_player and \
self.board.get(x,y+3) == self.current_player and \
self.board.get(x,y+4) == self.current_player:
return True
elif x + 4 < self.board.width and y + 4 < self.board.height and \
self.board.get(x,y) == self.current_player and \
self.board.get(x+1,y+1) == self.current_player and \
self.board.get(x+2,y+2) == self.current_player and \
self.board.get(x+3,y+3) == self.current_player and \
self.board.get(x+4,y+4) == self.current_player:
return True
elif x + 4 < self.board.width and y - 4 >= 0 and \
self.board.get(x,y) == self.current_player and \
self.board.get(x+1,y-1) == self.current_player and \
self.board.get(x+2,y-2) == self.current_player and \
self.board.get(x+3,y-3) == self.current_player and \
self.board.get(x+4,y-4) == self.current_player:
return True
return False
# 创建游戏对象并开始游戏
game = Game()
game.play()
```
请注意,这只是一个示例代码,可能需要进行更多的测试和改进,并根据需要进行自定义。
阅读全文
相关推荐



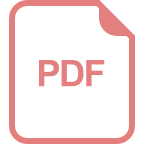
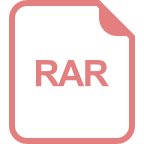
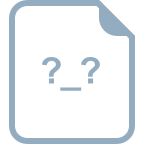
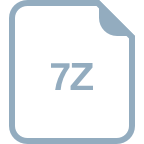
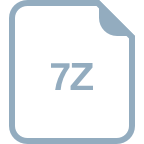
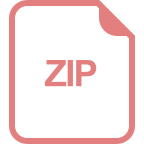