c++ mysqlpp库使用,插入语句
时间: 2024-10-23 19:03:48 浏览: 27
MySQL++库是一个C++接口,用于连接MySQL数据库并执行各种操作,包括数据插入。以下是使用MySQL++插入数据的基本步骤:
1. **包含头文件**:
首先,在你的源代码中引入`mysql++.h`和`mysql++.cpp`,这两个文件包含了MySQL++的函数声明和实现。
```cpp
#include <mysql++.h>
```
2. **建立连接**:
创建一个`MYSQL`对象,并使用`connect()`函数连接到你的MySQL服务器。
```cpp
MYSQL* conn;
conn = mysql_init(NULL);
if (!mysql_real_connect(conn, "localhost", "username", "password", "database_name", 0, NULL, 0))
{
// 处理错误
}
```
3. **准备SQL语句**:
使用`prepare()`方法准备插入语句。例如,如果你想要插入一条新用户记录,你可以这样做:
```cpp
std::string sql = "INSERT INTO users (name, email) VALUES (?, ?)";
```
4. **绑定参数**:
对于每个多余的问号(`?`),可以使用`bind()`方法将值绑定到占位符上。
```cpp
mysql_stmt* stmt = mysql_prepare(conn, sql.c_str());
if (!stmt)
{
// 处理错误
}
// 绑定参数,假设我们有名字"John Doe"和邮件"john.doe@example.com"
std::string name = "John Doe";
std::string email = "john.doe@example.com";
mysql_stmt_bind_param(stmt,:mysql::param_string(0), name.c_str()); // 第一个参数代表第一个?
mysql_stmt_bind_param(stmt,:mysql::param_string(1), email.c_str()); // 第二个参数代表第二个?
```
5. **执行插入**:
使用`execute()`方法执行SQL语句。
```cpp
int res = mysql_stmt_execute(stmt);
if (!res)
{
// 处理错误
}
```
6. **处理结果**:
如果需要,可以使用`affected_rows()`检查受影响的行数,关闭语句和连接等。
```cpp
unsigned long affected_rows = mysql_stmt_affected_rows(stmt);
mysql_free_result(mysql_stmt_result(stmt));
mysql_stmt_close(stmt);
mysql_close(conn);
```
阅读全文
相关推荐




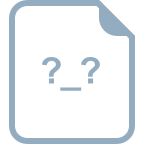



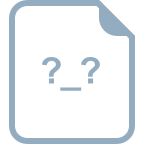






