def CountDigit(number, digit): sums = 0 for i in str(number): if i == str(digit): sums += 1 return sums
时间: 2024-05-23 18:13:46 浏览: 14
这是一个 Python 函数,用于统计一个整数中特定数字出现的次数。函数名为 CountDigit,有两个参数:number 表示要统计的整数,digit 表示要统计的数字。函数内部先将整数 number 转换成字符串,然后遍历字符串中的每个字符,如果字符等于参数 digit 表示的数字,则将计数器 sums 加 1。最后函数返回计数器 sums 的值,即数字 digit 在整数 number 中出现的次数。
相关问题
def CountDigit(number,digit): number1=abs(number) lst=list(str(number1)) s=0 for i in range(len(lst)): if str(digit) in lst: s+=1 return s
这段代码的函数定义是 `CountDigit(number, digit)`,接收两个参数 `number` 和 `digit`。它的功能是计算数字 `number` 中数字 `digit` 出现的次数。
代码实现的思路是将数字 `number` 转化为字符串形式,并将每一位存入一个列表中。然后遍历列表,统计数字 `digit` 在列表中出现的次数,最后返回次数值。
这段代码中存在一些问题:
1. `if str(digit) in lst` 应该改为 `if str(digit) in lst[i]`,表示判断数字 `digit` 是否在列表的第 `i` 个元素中出现;
2. 返回语句应该在循环之后,否则会在第一次循环时就结束函数并返回计数值。
下面是修改后的代码实现:
```python
def CountDigit(number, digit):
number1 = abs(number)
lst = list(str(number1))
s = 0
for i in range(len(lst)):
if str(digit) in lst[i]:
s += 1
return s
```
示例:
```python
>>> CountDigit(12345, 3)
1
>>> CountDigit(777, 7)
3
>>> CountDigit(10001, 0)
2
```
N=int(input()) for i in range(1,N+1): if '0' in str(i): continue if all(i % int(digit) == 0 for digit in str(i)): print(i)这个程序来求自除数哪里有错误
这个程序存在一个语法错误,即在第一行中没有使用任何变量名,程序无法运行。应该先声明一个变量N并将输入的值赋值给它,例如:
```
N = int(input())
```
此外,该程序有一个逻辑错误:当数字中包含0时,程序会跳过这个数字而不进行自除数的判断,这是不正确的。因为0除以任何数都是0,所以包含0的数字一定不是自除数。所以应该将第2行代码修改为:
```
if '0' in str(i):
continue
```
最终的程序应该是这样的:
```
N = int(input())
for i in range(1, N+1):
if '0' in str(i):
continue
if all(i % int(digit) == 0 for digit in str(i)):
print(i)
```
相关推荐
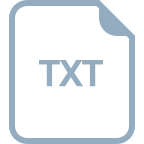
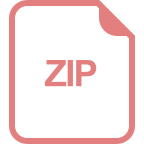













