Android 使RecyclerView轮播
时间: 2023-09-11 17:09:02 浏览: 149
要使用RecyclerView实现轮播效果,可以按照以下步骤操作:
1. 在布局文件中添加RecyclerView控件,设置宽高和其他属性:
```
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="200dp"
android:clipToPadding="false"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:paddingTop="8dp"
android:paddingBottom="8dp"
android:scrollbars="none"
app:layoutManager="androidx.recyclerview.widget.LinearLayoutManager"
app:orientation="horizontal" />
```
2. 创建一个Adapter类,并继承RecyclerView.Adapter<ViewHolder>,实现必要的方法,如onCreateViewHolder、onBindViewHolder和getItemCount等。
3. 在Adapter中添加一个getData()方法,用于获取数据源。
4. 在Activity或Fragment中,设置RecyclerView的Adapter和LayoutManager,并调用RecyclerView的smoothScrollToPosition()方法实现滚动效果:
```
private void initRecyclerView() {
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
layoutManager.setOrientation(LinearLayoutManager.HORIZONTAL);
recyclerView.setLayoutManager(layoutManager);
adapter = new MyAdapter();
recyclerView.setAdapter(adapter);
// 轮播效果
recyclerView.postDelayed(new Runnable() {
@Override
public void run() {
int position = (currentPosition + 1) % adapter.getData().size();
recyclerView.smoothScrollToPosition(position);
currentPosition = position;
recyclerView.postDelayed(this, 3000);
}
}, 3000);
}
```
5. 在轮播效果的实现中,通过RecyclerView的smoothScrollToPosition()方法实现滚动,同时设置一个延时任务,以达到循环轮播的效果。
6. 在延时任务中,更新当前的position,并调用RecyclerView的smoothScrollToPosition()方法实现滚动。
7. 在Adapter的getItemCount()方法中返回一个较大的值,以便实现循环滚动的效果:
```
@Override
public int getItemCount() {
return getData().size() * 1000;
}
```
注意:以上仅为简单的实现方式,实际应用中还需要考虑数据源的更新、点击事件的处理等问题。
阅读全文
相关推荐
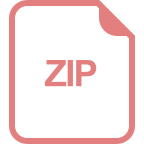


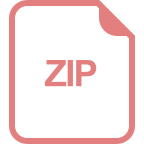
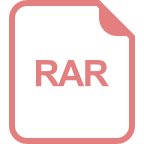
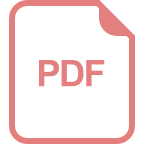
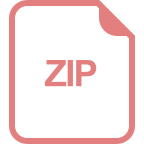
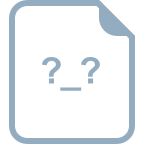
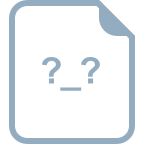
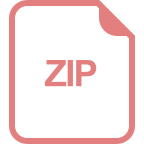
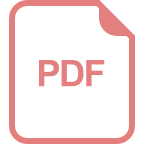
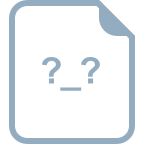
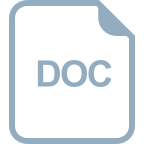
