C#电子体温单绘制
时间: 2023-07-12 22:18:57 浏览: 91
您好,以下是一个简单的 C# 代码示例,可以绘制出一个基本的电子体温单:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using System.Collections.Generic;
namespace TemperatureRecord
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
DrawTemperatureRecord();
}
private void DrawTemperatureRecord()
{
// 生成温度数据
List<double> temperatures = new List<double>();
for (double i = 0; i < 24; i += 0.5)
{
temperatures.Add(Math.Round(37.0 + (new Random().NextDouble() - 0.5) * 1.0, 1));
}
// 创建画布和画笔
Bitmap bmp = new Bitmap(pictureBox1.Width, pictureBox1.Height);
Graphics g = Graphics.FromImage(bmp);
Pen pen = new Pen(Color.Red, 2);
// 绘制温度曲线
int x0 = 60, y0 = 20;
int x1 = pictureBox1.Width - 40, y1 = pictureBox1.Height - 40;
int xInterval = (x1 - x0) / 48;
int yInterval = (y1 - y0) / 8;
for (int i = 0; i < temperatures.Count - 1; i++)
{
int x2 = x0 + (int)(xInterval * i);
int y2 = y0 + (int)((temperatures[i] - 35) * yInterval);
int x3 = x0 + (int)(xInterval * (i + 1));
int y3 = y0 + (int)((temperatures[i + 1] - 35) * yInterval);
g.DrawLine(pen, x2, y2, x3, y3);
}
// 绘制时间轴和温度轴
pen = new Pen(Color.Black, 1);
Font font = new Font("Arial", 8);
for (int i = 0; i <= 24; i += 2)
{
int x = x0 + i * xInterval;
g.DrawLine(pen, x, y0, x, y1);
g.DrawString(i.ToString(), font, Brushes.Black, x - 5, y1 + 5);
}
for (double i = 35; i <= 39; i += 0.5)
{
int y = y0 + (int)((i - 35) * yInterval);
g.DrawLine(pen, x0, y, x1, y);
g.DrawString(i.ToString(), font, Brushes.Black, x0 - 30, y - 5);
}
// 添加参考线和温度标记
pen = new Pen(Color.Gray, 1);
g.DrawLine(pen, x0, y0 + (int)((37 - 35) * yInterval), x1, y0 + (int)((37 - 35) * yInterval));
for (int i = 0; i < temperatures.Count; i++)
{
int x = x0 + (int)(xInterval * i);
int y = y0 + (int)((temperatures[i] - 35) * yInterval);
g.DrawLine(pen, x, y, x, y1);
g.DrawString(temperatures[i].ToString(), font, Brushes.Black, x - 5, y - 15);
}
// 显示画布
pictureBox1.Image = bmp;
}
}
}
```
这个代码会生成一个随机温度数据的电子体温单,其中包括时间轴、温度轴、参考线和温度标记。您可以根据需要自定义样式和数据。注意,这段代码是基于 Windows 窗体应用程序开发的,如果您使用的是其他类型的 C# 应用程序,可能需要对代码进行相应的修改。
相关推荐
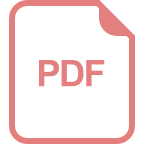














