@Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { // 获取当前线程的用户信息 Authentication authentication = SecurityContextHolder.getContext().getAuthentication(); String userId = authentication.getName(); String redisKey = "login:" + userId; LoginUser loginUser = JSON.parseObject(redisCache.getCacheObject(redisKey), LoginUser.class); String headerUrl = loginUser.getUser().getHeaderUrl(); System.out.println("获取到的图片路径为:" + headerUrl); if (loginUser != null && modelAndView != null){ modelAndView.addObject("loginUser",loginUser.getUser()); } } 对于以上代码,以下代码要写什么才能清空redis缓存, // 在模板引擎 TemplateEngine 之后执行 @Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { }
时间: 2023-12-17 16:02:23 浏览: 33
在 `afterCompletion` 方法中,可以通过 Redis Cache 的 `deleteObject` 方法清空缓存,示例代码如下:
```java
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception {
// 获取当前线程的用户信息
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
String userId = authentication.getName();
String redisKey = "login:" + userId;
redisCache.deleteObject(redisKey); // 清空缓存
}
```
相关问题
@Slf4j public class LoopCallInterceptor implements HandlerInterceptor { @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { //调用接口 // 头部获取当前请求所经过且没结束请求的path列表 // 判定path列表中是否包含当前path //无则正常访问 并记录到列表中 //有则告警循环调用,并终止调用,返回异常 return true; //return HandlerInterceptor.super.preHandle(request, response, handler); } @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { //HandlerInterceptor.super.postHandle(request, response, handler, modelAndView); //正常结束接口 //获取头部path列表 //从path列表中删除当前path } }
This is a Java class named `LoopCallInterceptor` that implements the `HandlerInterceptor` interface. The `HandlerInterceptor` interface is part of the Spring MVC framework and provides a way to intercept and handle requests and responses for Spring MVC applications.
The purpose of the `LoopCallInterceptor` class is to prevent circular or recursive calls to a Spring MVC controller method, which can cause infinite loops and consume excessive resources.
The `preHandle` method is called before a request is handled by a controller method. It takes in the `HttpServletRequest` and `HttpServletResponse` objects, as well as the `Object` representing the handler method that will handle the request.
The `preHandle` method first checks if the current request path is already present in the request header path list. If it is not present, it adds the current request path to the path list and returns `true` to indicate that the request can proceed normally. If it is already present, it logs a warning message for the circular or recursive call and returns `false` to indicate that the request should be stopped.
The `postHandle` method is called after a request has been handled by a controller method. It takes in the `HttpServletRequest`, `HttpServletResponse`, `Object` representing the handler method, and the `ModelAndView` object that contains the view and model data returned by the handler method.
The `postHandle` method removes the current request path from the request header path list.
Note that the `HandlerInterceptor` interface has three methods, but the `LoopCallInterceptor` class only implements the `preHandle` and `postHandle` methods. The `afterCompletion` method is called after the response has been rendered, but before the response has been committed.
@Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { // 获取当前线程的用户信息 Authentication authentication = SecurityContextHolder.getContext().getAuthentication(); String userId = authentication.getName(); String redisKey = "login:" + userId; LoginUser loginUser = JSON.parseObject(redisCache.getCacheObject(redisKey), LoginUser.class); String headerUrl = loginUser.getUser().getHeaderUrl(); System.out.println("获取到的图片路径为:" + headerUrl); if (loginUser != null && modelAndView != null){ modelAndView.addObject("loginUser",loginUser.getUser()); } } 对于以上代码,以下代码要写什么才能获得最新的redis, // 在模板引擎 TemplateEngine 之后执行 @Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { }
可以在afterCompletion方法中执行redis的刷新操作,使得模板引擎能够获取到最新的redis缓存数据。具体代码如下:
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception {
// 获取当前线程的用户信息
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
String userId = authentication.getName();
String redisKey = "login:" + userId;
LoginUser loginUser = JSON.parseObject(redisCache.getCacheObject(redisKey), LoginUser.class);
String headerUrl = loginUser.getUser().getHeaderUrl();
System.out.println("获取到的图片路径为:" + headerUrl);
// 刷新redis缓存
redisCache.refreshCache(redisKey);
}
相关推荐
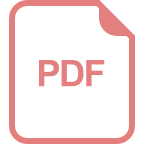
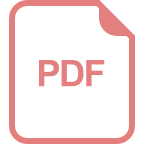
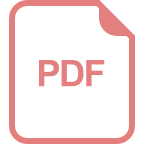













