(1)Student类包含学号、姓名、成绩、班级等字段(成员变量); (2)分别给字段定义自己的属性; (3)Student类中定义2个构造方法,其中一个是无参构造方法,另一个是接收4个参数的构造方法,分别用于为字段赋值; (4)定义成员方法,计算一个班级学生的最高成绩、最低成绩和平均成绩; (5)在主程序main()方法中分别调用不同的构造方法创建2个Student对象,并为属性赋值;并通过对象调用成员方法实现求一个班级学生的最高成绩、最低成绩和平均成绩的功能。
时间: 2023-11-04 22:32:37 浏览: 15
好的,以下是代码实现:
```python
class Student:
def __init__(self, stu_id='', name='', score=0, cls=''):
self.__stu_id = stu_id
self.__name = name
self.__score = score
self.__cls = cls
@property
def stu_id(self):
return self.__stu_id
@stu_id.setter
def stu_id(self, stu_id):
self.__stu_id = stu_id
@property
def name(self):
return self.__name
@name.setter
def name(self, name):
self.__name = name
@property
def score(self):
return self.__score
@score.setter
def score(self, score):
self.__score = score
@property
def cls(self):
return self.__cls
@cls.setter
def cls(self, cls):
self.__cls = cls
def calc_score(self, students):
max_score = max(s.score for s in students)
min_score = min(s.score for s in students)
avg_score = sum(s.score for s in students) / len(students)
return max_score, min_score, avg_score
```
在主程序中调用不同的构造方法创建2个Student对象,并为属性赋值,然后通过对象调用成员方法实现求一个班级学生的最高成绩、最低成绩和平均成绩的功能。
```python
if __name__ == '__main__':
stu1 = Student()
stu1.stu_id = '001'
stu1.name = 'Tom'
stu1.score = 80
stu1.cls = 'Class1'
stu2 = Student('002', 'Jerry', 90, 'Class1')
students = [stu1, stu2]
max_score, min_score, avg_score = stu1.calc_score(students)
print(f'Max score: {max_score}, Min score: {min_score}, Avg score: {avg_score}')
```
这样就可以实现求一个班级学生的最高成绩、最低成绩和平均成绩的功能了。
阅读全文
相关推荐
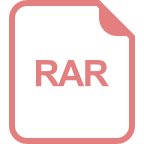
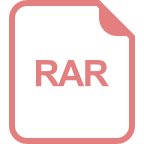
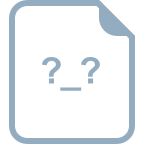

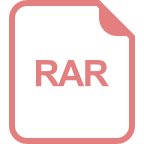
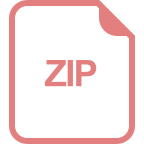












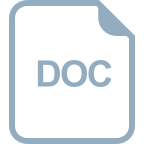
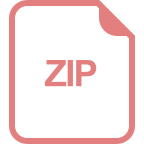