用c++写一个判断xyz点云是否有序的程序
时间: 2023-12-03 15:01:50 浏览: 38
好的,关于您的问题,可以考虑以下思路:对于给定的三维点云,我们可以对其进行排序来判断其是否有序。具体实现方法如下:
1. 定义一个结构体来存储三维点:
```
typedef struct {
float x;
float y;
float z;
} Point3D;
```
2. 读取点云数据,将所有点存储在一个数组中。
3. 对数组中的点进行排序。可以选择按照 x,y,z 坐标中的一个或多个进行排序,这里以按照 x 坐标为例,实现一个冒泡排序算法:
```
void bubbleSort(Point3D array[], int len) {
int i, j;
Point3D temp;
for (i = 0; i < len-1; i++) {
for (j = 0; j < len-1-i; j++) {
if (array[j].x > array[j+1].x) {
temp = array[j];
array[j] = array[j+1];
array[j+1] = temp;
}
}
}
}
```
4. 排序后,从头到尾遍历之前存储的点云数组,如果发现有任意一个点的 x 坐标不满足递增关系,则判断为无序点云,程序结束;否则全部遍历完之后判断为有序点云,程序结束。
完整代码如下:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct {
float x;
float y;
float z;
} Point3D;
void bubbleSort(Point3D array[], int len) {
int i, j;
Point3D temp;
for (i = 0; i < len-1; i++) {
for (j = 0; j < len-1-i; j++) {
if (array[j].x > array[j+1].x) {
temp = array[j];
array[j] = array[j+1];
array[j+1] = temp;
}
}
}
}
int main() {
Point3D point_cloud[10] = {{0,1,3},{1,2,3},{3,2,1},{0,0,0},{0,1,1},{1,2,2},{0,0,1},{2,2,3},{3,3,3},{1,1,1}};
bubbleSort(point_cloud, 10);
int i = 0;
for (; i < 9; i++) {
if (point_cloud[i].x > point_cloud[i+1].x) {
printf("The point cloud is not ordered.\n");
return 0;
}
}
printf("The point cloud is ordered.\n");
return 0;
}
```
注意:这里给定了一个点云数组 point_cloud,只是一个用于测试的样例数据,实际应用中需要根据具体情况修改代码。
相关推荐
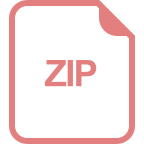














