pycharm cannot import name 'GridSearchC' from 'sklearn.model_selection'
时间: 2023-08-15 16:03:08 浏览: 163
这个错误通常表示你需要更新 scikit-learn 库。你可以通过运行以下命令来更新库:
```
pip install -U scikit-learn
```
如果你已经使用了虚拟环境,请确保在激活虚拟环境后运行此命令。如果更新库还是无法解决问题,请检查你的代码中是否有其他依赖库与 scikit-learn 版本不兼容。
相关问题
pycharm报错from sklearn.model_selection import train_test_split ModuleNotFoundError: No module named 'sklearn'
这个错误提示是因为你的 PyCharm 环境中没有安装 scikit-learn(sklearn)模块。你可以通过以下命令使用 pip 安装:
```
pip install -U scikit-learn
```
如果你使用的是 Anaconda 环境,可以使用以下命令安装:
```
conda install scikit-learn
```
安装完成后,再次运行代码应该就没有问题了。
# This is a sample Python script. # Press Shift+F10 to execute it or replace it with your code. # Press Double Shift to search everywhere for classes, files, tool windows, actions, and settings. def print_hi(name): # Use a breakpoint in the code line below to debug your script. print(f'Hi, {name}') # Press Ctrl+F8 to toggle the breakpoint. # Press the green button in the gutter to run the script. if __name__ == '__main__': print_hi('PyCharm') # See PyCharm help at https://www.jetbrains.com/help/pycharm/ from sklearn.datasets import load_iris from sklearn.linear_model import LogisticRegression from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score iris = load_iris() X = iris.data y = iris.target X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42) # 使用 'ovr' 多分类方法 model1 = LogisticRegression(multi_class='ovr', solver='liblinear') model1.fit(X_train, y_train) y_pred1 = model1.predict(X_test) acc1 = accuracy_score(y_test, y_pred1) print('Accuracy score using "ovr" method:', acc1)修改该程序使上述程序结果数据可视化
要进行结果数据的可视化,您需要使用某个绘图库。这里我们选择 matplotlib 库。为了可视化结果,您需要将分类结果投影到一个二维平面上。这里我们使用主成分分析(PCA)来实现。
下面是修改后的代码,它将分类结果投影到二维平面上,并绘制出不同类别的数据点:
``` python
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from sklearn.decomposition import PCA
# 加载数据
iris = load_iris()
X = iris.data
y = iris.target
# 进行主成分分析
pca = PCA(n_components=2)
X_pca = pca.fit_transform(X)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X_pca, y, test_size=0.3, random_state=42)
# 训练模型并进行预测
model = LogisticRegression(multi_class='ovr', solver='liblinear')
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
acc = accuracy_score(y_test, y_pred)
print('Accuracy score using "ovr" method:', acc)
# 绘制分类结果
x_min, x_max = X_pca[:, 0].min() - .5, X_pca[:, 0].max() + .5
y_min, y_max = X_pca[:, 1].min() - .5, X_pca[:, 1].max() + .5
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.02), np.arange(y_min, y_max, 0.02))
Z = model.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.figure(1, figsize=(4, 3))
plt.contourf(xx, yy, Z, cmap=plt.cm.RdYlBu)
plt.scatter(X_pca[:, 0], X_pca[:, 1], c=y, cmap=plt.cm.RdYlBu)
plt.xlabel('PC1')
plt.ylabel('PC2')
plt.show()
```
运行该程序,您将看到一个分类结果的可视化图像,其中不同颜色的区域表示不同的分类结果。您可以通过观察分类结果图像来了解模型的分类效果。
阅读全文
相关推荐
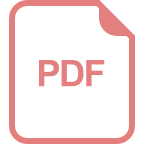
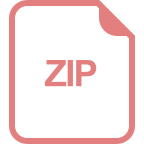
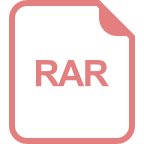
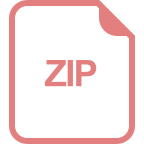
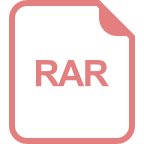
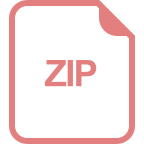
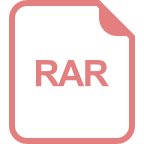
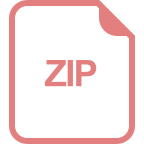
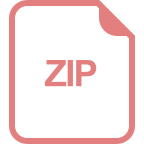
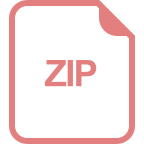
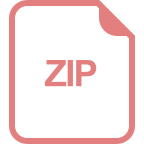
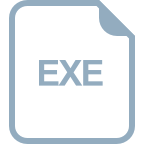
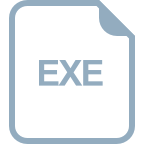
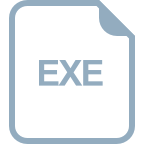