基于51单片机的数字温度传感器的数字温度计怎么连线
时间: 2023-07-05 10:16:34 浏览: 182
基于51单片机的数字温度传感器通常会采用DS18B20数字温度传感器。下面是DS18B20数字温度传感器与51单片机的连接方式:
1. 将DS18B20数字温度传感器的VDD引脚连接到单片机的3.3V电源引脚或5V电源引脚。
2. 将DS18B20数字温度传感器的GND引脚连接到单片机的GND引脚。
3. 将DS18B20数字温度传感器的DQ引脚连接到单片机的任意一个IO口,该IO口需要配置为开漏输出模式。
需要注意的是,DS18B20数字温度传感器是一种单总线设备,因此在连接时需要注意单总线的拓扑结构。可以参考下面的连接图示进行连接:

相关问题
基于51单片机的数字温度传感器的数字温度计
以下是基于51单片机的数字温度传感器的数字温度计代码,使用的是DS18B20数字温度传感器:
```c
#include <reg51.h>
#include <stdio.h>
#define DQ P3_4
typedef unsigned char uchar;
typedef unsigned int uint;
sbit RS=P1^0;
sbit RW=P1^1;
sbit E=P1^2;
sbit DQ=P3^4;
void delay(uint i) {
uint j;
for (; i > 0; i--) {
for (j = 112; j > 0; j--);
}
}
void init_DS18B20() {
uchar i;
DQ = 1;
delay(1);
DQ = 0;
delay(500);
DQ = 1;
delay(60);
i = DQ;
delay(500);
}
uchar read_DS18B20() {
uchar i, j, k;
uchar temp = 0x00;
for (j = 8; j > 0; j--) {
DQ = 0;
delay(1);
DQ = 1;
delay(1);
k = DQ;
i = DQ;
delay(1);
temp = (temp >> 1) | (k << 7);
}
return temp;
}
void write_DS18B20(uchar dat) {
uchar j, testb;
for (j = 8; j > 0; j--) {
testb = dat & 0x01;
dat = dat >> 1;
if (testb) {
DQ = 0;
delay(1);
DQ = 1;
delay(60);
} else {
DQ = 0;
delay(60);
DQ = 1;
delay(1);
}
}
}
uchar get_temp() {
uchar a, b;
init_DS18B20();
write_DS18B20(0xcc);
write_DS18B20(0x44);
delay(100);
init_DS18B20();
write_DS18B20(0xcc);
write_DS18B20(0xbe);
a = read_DS18B20();
b = read_DS18B20();
return a;
}
void LCD_Write_Com(uchar com) {
RS = 0;
RW = 0;
P2 = com;
delay(5);
E = 1;
delay(5);
E = 0;
}
void LCD_Write_Data(uchar dat) {
RS = 1;
RW = 0;
P2 = dat;
delay(5);
E = 1;
delay(5);
E = 0;
}
void LCD_Init() {
LCD_Write_Com(0x38);
LCD_Write_Com(0x0c);
LCD_Write_Com(0x06);
LCD_Write_Com(0x01);
}
void main() {
uchar temp;
LCD_Init();
while (1) {
temp = get_temp();
LCD_Write_Com(0x80);
printf("Temperature:");
LCD_Write_Com(0x8d);
printf("%d", temp);
delay(500);
}
}
```
以上代码通过初始化DS18B20数字温度传感器、读取传感器数据、将数据显示在LCD1602液晶显示屏上的方式实现了数字温度计的功能。其中,使用了定时器延时函数`delay()`和串口通信函数`printf()`。需要注意的是,需要将DS18B20数字温度传感器连接到51单片机的P3.4引脚,LCD1602液晶显示屏连接到P2口。
基于51单片机的数字温度传感器的数字温度计代码
下面是基于51单片机的数字温度传感器的数字温度计代码,主要包括初始化DS18B20传感器、读取温度值等功能。
```c
#include <reg51.h>
#include <stdio.h>
#define DQ P3_4 //定义DQ引脚
sbit LED = P1^0; //定义LED引脚
//延时函数
void Delay1us()
{
unsigned char i;
for(i=0;i<12;i++);
}
//DS18B20初始化函数
void Init_DS18B20()
{
DQ = 1; //DQ引脚输出1
Delay1us();
DQ = 0; //DQ引脚输出0
Delay1us();
DQ = 1; //DQ引脚输出1
Delay1us();
}
//DS18B20发送一个字节函数
void WriteByte_DS18B20(unsigned char dat)
{
unsigned char i;
for(i=0;i<8;i++)
{
DQ = 0; //DQ引脚输出0
Delay1us();
DQ = dat&0x01; //将dat的最低位发送出去
Delay1us();
DQ = 1; //DQ引脚输出1
Delay1us();
dat >>= 1; //dat右移一位
}
}
//DS18B20读取一个字节函数
unsigned char ReadByte_DS18B20()
{
unsigned char dat = 0;
unsigned char i;
for(i=0;i<8;i++)
{
DQ = 0; //DQ引脚输出0
Delay1us();
DQ = 1; //DQ引脚输出1
Delay1us();
dat >>= 1; //dat右移一位
if(DQ) dat |= 0x80; //如果DQ引脚为1,则dat最高位为1
Delay1us();
}
return dat;
}
//DS18B20温度转换函数
void Convert_DS18B20()
{
Init_DS18B20(); //初始化DS18B20传感器
WriteByte_DS18B20(0xCC); //跳过ROM操作
WriteByte_DS18B20(0x44); //启动温度转换
}
//DS18B20读取温度函数
float ReadTemp_DS18B20()
{
unsigned char TH, TL;
int temp;
float temp_value;
Init_DS18B20(); //初始化DS18B20传感器
WriteByte_DS18B20(0xCC); //跳过ROM操作
WriteByte_DS18B20(0xBE); //读取温度寄存器
TL = ReadByte_DS18B20(); //读取温度低8位
TH = ReadByte_DS18B20(); //读取温度高8位
temp = TH;
temp <<= 8;
temp |= TL; //将温度高8位和低8位组合成16位温度值
temp_value = temp*0.0625; //转换为浮点数
return temp_value;
}
void main()
{
float temp;
while(1)
{
Convert_DS18B20(); //启动温度转换
Delay1us();
temp = ReadTemp_DS18B20(); //读取温度值
printf("Temperature is: %.1f\n", temp); //通过串口输出温度值
if(temp > 25) //如果温度大于25度,LED闪烁
{
LED = 1;
Delay1us();
LED = 0;
Delay1us();
}
else
{
LED = 0;
}
Delay1us(500000);
}
}
```
注意:该代码中使用了串口输出函数printf(),需要另外添加串口初始化函数,并连接好51单片机的串口引脚。同时,需要根据实际情况修改LED引脚的定义。
阅读全文
相关推荐
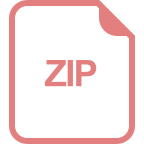
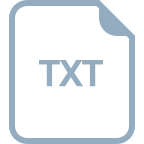

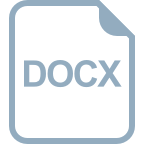
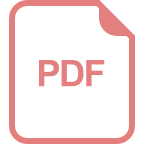
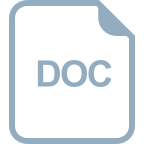
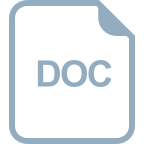
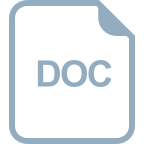
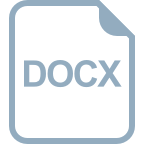
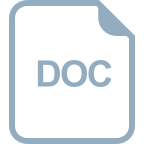
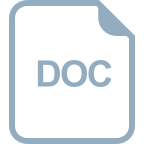
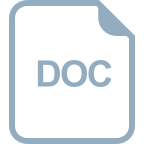
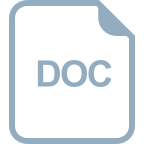
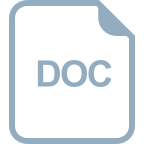