byte[] bytes = cipher.doFinal(str.getBytes("utf-8")); String result = new BASE64Encoder().encode(bytes); result = result.toString().replaceAll("\r\n", ""); result = result.replaceAll("\\+", ADD); return result;
时间: 2023-10-31 09:04:27 浏览: 33
这段代码的作用是将字符串进行加密,并返回加密后的结果。首先,使用指定的加密算法对字符串进行加密操作。然后,将加密后的结果转换为字节数组。接下来,将字节数组使用Base64编码转换为字符串。最后,对字符串进行一些替换操作,去除换行符并将加号替换为ADD。最终,返回加密后的结果字符串。
请注意,代码中的ADD是一个变量,需要提前定义。另外,这段代码使用了Java标准库中的一些类和方法,需要先导入相应的包。
相关问题
将以下JAVA代码转为PHP代码:import javax.crypto.Cipher; import javax.crypto.spec.SecretKeySpec; import java.util.Base64; public class AesTool { public static String encrypt(String str, String key) throws Exception { Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding"); SecretKeySpec keySpec = new SecretKeySpec(key.getBytes("UTF-8"), "AES"); cipher.init(Cipher.ENCRYPT_MODE, keySpec); byte[] result = cipher.doFinal(str.getBytes("UTF-8")); return Base64.getEncoder().encodeToString(result); } public static String decrypt(String base64Str, String key) throws Exception { Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding"); SecretKeySpec keySpec = new SecretKeySpec(key.getBytes("UTF-8"), "AES"); cipher.init(Cipher.DECRYPT_MODE, keySpec); byte[] decoded = cipher.doFinal(Base64.getDecoder().decode(base64Str)); return new String(decoded, "UTF-8"); } }
以下是将JAVA代码转换为PHP代码的示例:
```php
<?php
class AesTool {
public static function encrypt($str, $key) {
$cipher = "AES-128-ECB";
$ivlen = openssl_cipher_iv_length($cipher);
$iv = openssl_random_pseudo_bytes($ivlen);
$result = openssl_encrypt($str, $cipher, $key, OPENSSL_RAW_DATA, $iv);
return base64_encode($result);
}
public static function decrypt($base64Str, $key) {
$cipher = "AES-128-ECB";
$base64Str = base64_decode($base64Str);
$ivlen = openssl_cipher_iv_length($cipher);
$iv = openssl_random_pseudo_bytes($ivlen);
$decoded = openssl_decrypt($base64Str, $cipher, $key, OPENSSL_RAW_DATA, $iv);
return $decoded;
}
}
```
在这个PHP代码示例中,我们使用了openssl扩展来实现AES算法的加解密,使用的是128位密钥和ECB模式,并且使用PKCS5Padding对明文进行了补位。
public static String encryptRSA(String encodeValue) { String decryptValue = ""; try { String privateKey = CryptoRsaKey_NEO.getPrivateKey(); RSAPrivateKey privateKey1 = (RSAPrivateKey)getPrivateKeyDec(privateKey); byte[] decBytesBase64 = encodeValue.getBytes(); Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding"); cipher.init(Cipher.DECRYPT_MODE, privateKey1); byte[] resultBytes = cipher.doFinal(decBytesBase64); String aaa = new String(resultBytes,"UTF-8"); String bbb = Arrays.toString(resultBytes); System.out.println("Base64‘OaaaF" + aaa); System.out.println("Base64‘ObbbF" + bbb); decryptValue = new String(java.util.Base64.getEncoder().encode(resultBytes)); } catch (Exception e) { WPLErrUtil.logger("CipherUtil", "decryptRSA", "RSA" + encodeValue + "," + e.getMessage() + ":" + e.toString()); return null; } return decryptValue; }请根据上面的解密方法写出对应的加密方法
根据上面的解密方法,可以编写对应的加密方法如下:
```
public static String encryptRSA(String plainValue) {
String encryptValue = "";
try {
String publicKey = CryptoRsaKey_NEO.getPublicKey();
RSAPublicKey publicKey1 = (RSAPublicKey)getPublicKeyDec(publicKey);
byte[] plainBytes = plainValue.getBytes("UTF-8");
Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
cipher.init(Cipher.ENCRYPT_MODE, publicKey1);
byte[] resultBytes = cipher.doFinal(plainBytes);
encryptValue = java.util.Base64.getEncoder().encodeToString(resultBytes);
} catch (Exception e) {
WPLErrUtil.logger("CipherUtil", "encryptRSA", "RSA" + plainValue + "," + e.getMessage() + ":" + e.toString());
return null;
}
return encryptValue;
}
```
这个方法接收一个字符串参数作为明文,返回一个字符串作为密文。它使用了与解密方法中相同的公钥和填充方式,然后将明文转换为字节数组,使用公钥进行加密,并将结果字节数组转换为Base64编码的字符串。
相关推荐
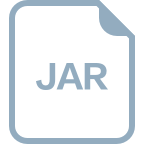
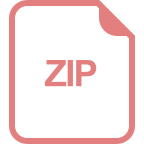












