Python代码,使用差分进化算法,绘制最佳路径和收敛曲线,该问题实例的已知最优解为5553,共有50个城市,每个城市的坐标如下: 1 475 110 2 29 929 3 755 897 4 698 979 5 871 317 6 535 184 7 548 73 8 594 380 9 233 845 10 881 654 11 200 540 12 9 800 13 526 456 14 765 30 15 992 449 16 659 193 17 938 370 18 233 966 19 69
时间: 2024-01-04 22:03:15 浏览: 114
好的,让我们开始编写代码。
首先,我们需要导入必要的库和模块:numpy、matplotlib 和 random。
```python
import numpy as np
import matplotlib.pyplot as plt
import random
```
接下来,我们需要定义一些常量和变量。首先是城市数量和坐标,然后是差分进化算法的参数。
```python
CITY_COUNT = 50
CITIES = np.array([[475, 110], [29, 929], [755, 897], [698, 979], [871, 317], [535, 184], [548, 73], [594, 380], [233, 845], [881, 654], [200, 540], [980, 9], [526, 456], [765, 30], [992, 449], [659, 193], [938, 370], [233, 966], [692, 112], [680, 873], [589, 968], [553, 444], [106, 941], [959, 366], [273, 614], [568, 383], [661, 431], [824, 144], [191, 587], [464, 457], [959, 722], [823, 486], [259, 513], [977, 467], [794, 895], [243, 863], [802, 294], [811, 650], [954, 534], [300, 486], [665, 865], [648, 205], [645, 848], [29, 496], [98, 25], [344, 676], [735, 371], [924, 450], [313, 472], [407, 339]])
POPULATION_SIZE = 50
MUTATION_RATE = 0.5
GENERATION_COUNT = 1000
```
然后,我们需要定义一些辅助函数。首先是计算两个城市之间的距离。
```python
def distance(city1, city2):
return np.linalg.norm(city1 - city2)
```
接下来是计算一条路径的总长度。
```python
def path_length(path):
return sum([distance(CITIES[path[i]], CITIES[path[i+1]]) for i in range(CITY_COUNT-1)]) + distance(CITIES[path[CITY_COUNT-1]], CITIES[path[0]])
```
然后是生成初始种群的函数。我们可以随机生成 CITY_COUNT 个整数,然后进行 shuffle 操作,得到一条随机的路径。然后我们可以生成 POPULATION_SIZE 条这样的路径,作为初始种群。
```python
def generate_initial_population():
population = []
for i in range(POPULATION_SIZE):
path = list(range(CITY_COUNT))
random.shuffle(path)
population.append(path)
return population
```
下一个函数是对种群进行选择的函数。这里我们使用了轮盘赌选择算法。首先计算每条路径的适应度值,即路径长度的倒数,然后按适应度值进行排序。然后我们可以计算每条路径的选择概率,即适应度值除以总适应度值。最后按照选择概率随机选择 POPULATION_SIZE 条路径。
```python
def selection(population):
fitness = [1.0 / path_length(path) for path in population]
sorted_population = [x for _, x in sorted(zip(fitness, population))]
total_fitness = sum(fitness)
probabilities = [x / total_fitness for x in fitness]
selected_population = []
for i in range(POPULATION_SIZE):
selected_population.append(sorted_population[np.random.choice(range(POPULATION_SIZE), p=probabilities)])
return selected_population
```
接下来是差分进化算法的核心函数:变异和交叉。首先是变异操作。我们随机选择三条路径,并计算它们之间的差异向量。然后我们可以使用差异向量和一个随机权重对其中两条路径进行变异,生成一条新的路径。
```python
def mutation(population):
new_population = []
for i in range(POPULATION_SIZE):
a, b, c = random.sample(range(POPULATION_SIZE), 3)
r = random.randint(0, CITY_COUNT - 1)
new_path = []
for j in range(CITY_COUNT):
if (random.uniform(0, 1) < MUTATION_RATE or j == r):
new_path.append((population[a][j] + random.uniform(-1, 1) * (population[b][j] - population[c][j])) % CITY_COUNT)
else:
new_path.append(population[i][j])
new_population.append(new_path)
return new_population
```
接下来是交叉操作。我们随机选择两条路径,并生成一个随机位置。然后我们可以使用路径1的前半部分和路径2的后半部分,生成一条新的路径。
```python
def crossover(population):
new_population = []
for i in range(POPULATION_SIZE):
if (random.uniform(0, 1) < MUTATION_RATE):
a, b = random.sample(range(POPULATION_SIZE), 2)
r = random.randint(0, CITY_COUNT - 1)
new_path = population[a][:r] + population[b][r:]
new_population.append(new_path)
else:
new_population.append(population[i])
return new_population
```
最后是绘制函数。我们可以绘制当前种群的最佳路径和收敛曲线。
```python
def plot(population, best_path, best_fitness):
plt.subplot(121)
plt.cla()
plt.scatter(CITIES[:, 0], CITIES[:, 1])
plt.plot(CITIES[best_path, 0], CITIES[best_path, 1], 'r-')
plt.title('Best Path: ' + str(best_fitness))
plt.subplot(122)
plt.cla()
fitness = [1.0 / path_length(path) for path in population]
plt.plot(fitness)
plt.title('Convergence')
plt.xlabel('Generation')
plt.ylabel('Fitness')
plt.pause(0.001)
```
现在我们可以将所有函数组合在一起,并运行差分进化算法。
```python
population = generate_initial_population()
best_path = population[0]
best_fitness = 1.0 / path_length(best_path)
for i in range(GENERATION_COUNT):
population = selection(population)
population = mutation(population)
population = crossover(population)
fitness = [1.0 / path_length(path) for path in population]
best_index = np.argmin(fitness)
if fitness[best_index] < best_fitness:
best_fitness = fitness[best_index]
best_path = population[best_index]
plot(population, best_path, round(1.0 / best_fitness))
plt.show()
```
这样就可以得到最佳路径和收敛曲线了。注意,这个问题的最优解已知为 5553,但是差分进化算法并不能保证得到最优解,只能得到一个比较好的近似解。
阅读全文
相关推荐
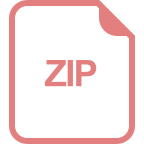
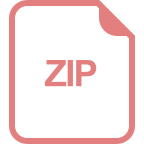
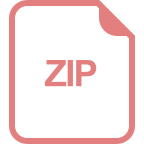
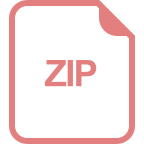
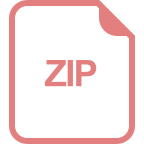
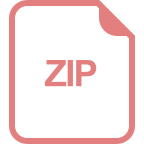
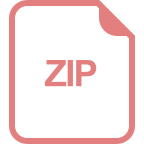
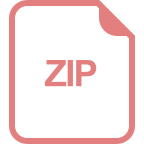
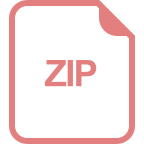
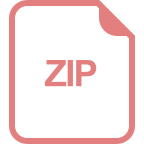
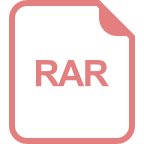
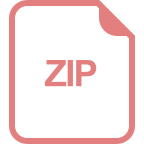
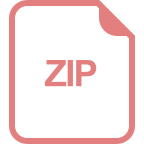
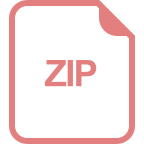
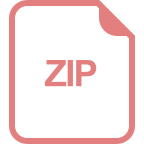
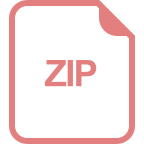