基于HTML+CSS+JavaScript制作网页设计案例25违停查询
时间: 2023-08-09 20:04:01 浏览: 541
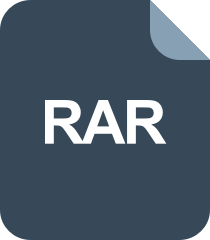
html css javascript web网页设计案例大全

以下是一个基于HTML+CSS+JavaScript制作的网页设计案例25违停查询:
1. HTML代码
在HTML代码中,我们使用了一个简单的表单,让用户能够输入车牌号和查询日期进行查询。
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>违停查询</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<h1>违停查询</h1>
<form>
<label for="plateNum">车牌号:</label>
<input type="text" id="plateNum" name="plateNum" required>
<label for="queryDate">查询日期:</label>
<input type="date" id="queryDate" name="queryDate" required>
<button type="submit" id="queryBtn">查询</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
```
2. CSS代码
在CSS代码中,我们设置了页面的样式,包括背景颜色、字体样式、输入框和按钮的样式等等。
```css
body {
background-color: #f2f2f2;
font-family: Arial, sans-serif;
}
.container {
margin: 50px auto;
width: 500px;
background-color: #fff;
padding: 30px;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.3);
}
h1 {
text-align: center;
margin-bottom: 30px;
}
form {
display: flex;
flex-direction: column;
}
label {
margin-bottom: 10px;
}
input[type="text"],
input[type="date"] {
padding: 10px;
margin-bottom: 20px;
border-radius: 5px;
border: none;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.3);
}
button {
background-color: #0099ff;
color: #fff;
padding: 10px;
border: none;
border-radius: 5px;
cursor: pointer;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.3);
}
button:hover {
background-color: #0077cc;
}
```
3. JavaScript代码
在JavaScript代码中,我们使用了AJAX技术,向服务器发送查询请求,并且在页面上显示查询结果。
```javascript
const form = document.querySelector('form');
const queryBtn = document.querySelector('#queryBtn');
queryBtn.addEventListener('click', function(event) {
event.preventDefault();
const plateNum = document.querySelector('#plateNum').value;
const queryDate = document.querySelector('#queryDate').value;
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
showResults(response);
}
}
const url = `https://example.com/api/query?plateNum=${plateNum}&queryDate=${queryDate}`;
xhr.open('GET', url, true);
xhr.send();
});
function showResults(response) {
const table = document.createElement('table');
const thead = document.createElement('thead');
const tbody = document.createElement('tbody');
const headRow = document.createElement('tr');
const dateHead = document.createElement('th');
const timeHead = document.createElement('th');
const locationHead = document.createElement('th');
const violationHead = document.createElement('th');
const fineHead = document.createElement('th');
dateHead.textContent = '日期';
timeHead.textContent = '时间';
locationHead.textContent = '地点';
violationHead.textContent = '违停条例';
fineHead.textContent = '罚款金额';
headRow.appendChild(dateHead);
headRow.appendChild(timeHead);
headRow.appendChild(locationHead);
headRow.appendChild(violationHead);
headRow.appendChild(fineHead);
thead.appendChild(headRow);
response.forEach(record => {
const row = document.createElement('tr');
const dateCell = document.createElement('td');
const timeCell = document.createElement('td');
const locationCell = document.createElement('td');
const violationCell = document.createElement('td');
const fineCell = document.createElement('td');
dateCell.textContent = record.date;
timeCell.textContent = record.time;
locationCell.textContent = record.location;
violationCell.textContent = record.violation;
fineCell.textContent = record.fine;
row.appendChild(dateCell);
row.appendChild(timeCell);
row.appendChild(locationCell);
row.appendChild(violationCell);
row.appendChild(fineCell);
tbody.appendChild(row);
});
table.appendChild(thead);
table.appendChild(tbody);
const container = document.querySelector('.container');
container.appendChild(table);
}
```
总之,这个网页设计案例着重强调了简单明了和易于使用。用户可以轻松地输入车牌号和查询日期,然后点击“查询”按钮,查询结果也是易于阅读和理解的。同时,网页还使用了AJAX技术,让用户能够在不刷新页面的情况下获得查询结果。
阅读全文
相关推荐
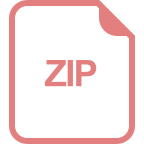
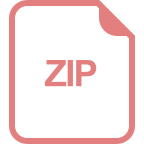
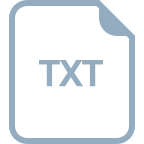
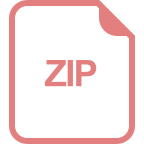
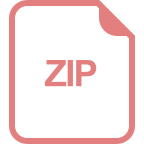
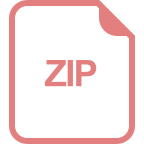
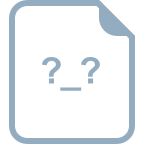
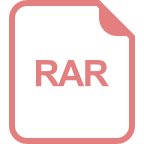
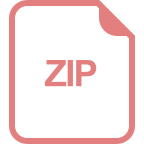
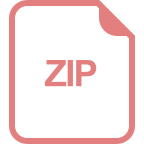
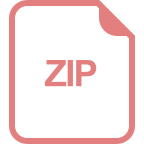
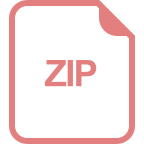
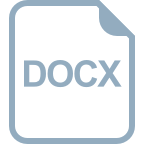
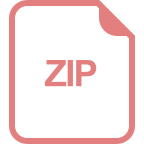
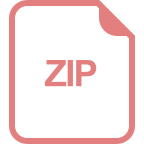
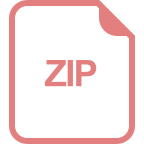