根据一部分求第二部分代码,头文件源文件分别标出
时间: 2024-10-25 07:11:00 浏览: 11
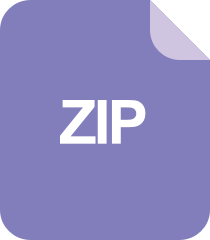
c代码-第三课作业 第一部分
根据提供的文档内容,以下是 `Box` 类的设计和实现的详细代码:
### 头文件 (Box.h)
```cpp
#ifndef BOX_H
#define BOX_H
template <typename T>
class Box {
public:
// 构造函数
Box(T length = 0, T width = 0, T height = 0) : length(length), width(width), height(height) {}
// 获取属性的方法
T getLength() const { return length; }
T getWidth() const { return width; }
T getHeight() const { return height; }
// 设置属性的方法
void setBox(T length, T width, T height);
void setBox(T size);
void setBox(const Box<T>& b);
// 运算符重载
bool operator==(const Box<T>& b) const;
Box<T> operator+(const Box<T>& b) const;
private:
T length;
T width;
T height;
};
// 实现 SetBox 方法
template <typename T>
void Box<T>::setBox(T length, T width, T height) {
this->length = length;
this->width = width;
this->height = height;
}
template <typename T>
void Box<T>::setBox(T size) {
this->length = size;
this->width = size;
this->height = size;
}
template <typename T>
void Box<T>::setBox(const Box<T>& b) {
this->length = b.length;
this->width = b.width;
this->height = b.height;
}
// 实现运算符重载
template <typename T>
bool Box<T>::operator==(const Box<T>& b) const {
return (this->length == b.length && this->width == b.width && this->height == b.height);
}
template <typename T>
Box<T> Box<T>::operator+(const Box<T>& b) const {
return Box<T>(this->length + b.length, this->width + b.width, this->height + b.height);
}
#endif // BOX_H
```
### 源文件 (main.cpp)
```cpp
#include <iostream>
#include "Box.h"
int main() {
// 创建 Box 对象并初始化
Box<int> box1(10, 20, 30);
Box<int> box2(5, 15, 25);
// 输出初始值
std::cout << "Box1: Length=" << box1.getLength() << ", Width=" << box1.getWidth() << ", Height=" << box1.getHeight() << std::endl;
std::cout << "Box2: Length=" << box2.getLength() << ", Width=" << box2.getWidth() << ", Height=" << box2.getHeight() << std::endl;
// 使用 setBox 方法
box1.setBox(15, 25, 35);
std::cout << "After setting Box1: Length=" << box1.getLength() << ", Width=" << box1.getWidth() << ", Height=" << box1.getHeight() << std::endl;
// 使用 setBox(size) 方法
box1.setBox(20);
std::cout << "After setting Box1 to a square: Length=" << box1.getLength() << ", Width=" << box1.getWidth() << ", Height=" << box1.getHeight() << std::endl;
// 使用 setBox(Box b) 方法
box1.setBox(box2);
std::cout << "After copying Box2 to Box1: Length=" << box1.getLength() << ", Width=" << box1.getWidth() << ", Height=" << box1.getHeight() << std::endl;
// 使用运算符重载
if (box1 == box2) {
std::cout << "Box1 and Box2 are equal." << std::endl;
} else {
std::cout << "Box1 and Box2 are not equal." << std::endl;
}
Box<int> box3 = box1 + box2;
std::cout << "Box3 (Box1 + Box2): Length=" << box3.getLength() << ", Width=" << box3.getWidth() << ", Height=" << box3.getHeight() << std::endl;
return 0;
}
```
### 总结
- **头文件 (`Box.h`)**: 定义了 `Box` 类,包括属性、方法和运算符重载。
- **源文件 (`main.cpp`)**: 包含主函数,用于测试 `Box` 类的各种功能,包括属性的设置和获取、运算符重载等。
通过以上代码,可以验证 `Box` 类的功能,并确保其正确性和灵活性。
阅读全文
相关推荐
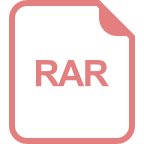
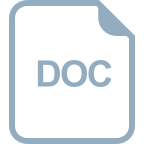
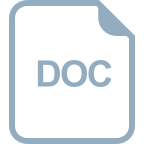
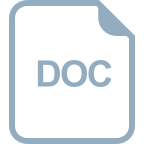
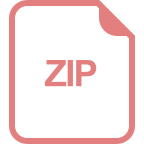
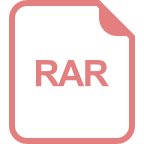
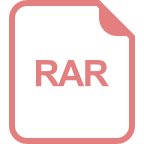
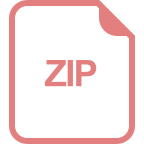
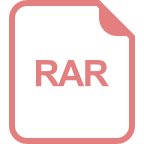
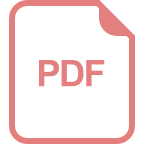
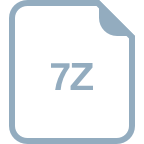
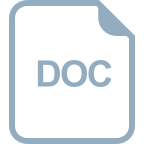
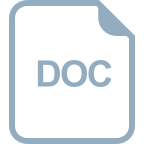
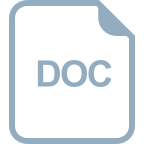
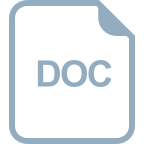
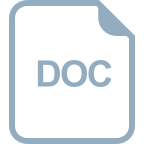
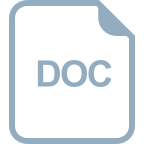
