让C++程序分别读入两个十进制数M、N,将十进制数M转换成N进制的数。
时间: 2024-04-09 19:29:14 浏览: 14
好的!要实现将十进制数M转换为N进制的数,您可以使用C++中的循环和除法取余运算。
以下是一个示例代码:
```cpp
#include <iostream>
#include <string>
#include <stack>
std::string convertToBase(int num, int base) {
std::stack<char> digits;
while (num > 0) {
int remainder = num % base;
if (remainder < 10) {
digits.push(remainder + '0'); // 将余数转换为字符并入栈
} else {
digits.push(remainder - 10 + 'A'); // 将余数转换为大写字母并入栈
}
num /= base;
}
std::string result;
while (!digits.empty()) {
result += digits.top(); // 从栈中取出字符并拼接到结果字符串
digits.pop();
}
return result;
}
int main() {
int M, N;
std::cout << "请输入两个十进制数:" << std::endl;
std::cin >> M >> N;
std::string convertedNum = convertToBase(M, N);
std::cout << "转换后的结果为:" << convertedNum << std::endl;
return 0;
}
```
以上代码中,`convertToBase` 函数用于将十进制数转换为指定进制的字符串。它使用了一个栈来保存每一位的数字或字母,并将它们逆序拼接成最终的结果字符串。
请注意,此代码假设输入的数值是有效的,并且没有进行错误处理。您可以根据实际需求进行修改和优化。
相关推荐
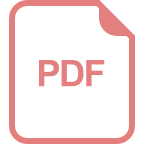
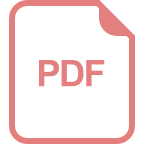
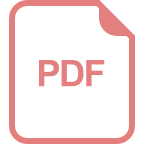














