自定义类模拟三维向量及其运算定义一个三维向量类,并定义相应的特殊方法实现:1)两个该类对象的加、减运算(要求支持运算符+、-);2)实现该类对象与标量的乘、除运算(要求支持运算符*、/,乘法时包括标量在前和后两种情况);3)以及向量的print方法; 向量的长度的定义;向量的点积以及叉积的定义; 定义一个类属性,表征已经实例化的对象的个数。程序必须规范,增加必要的注释; 在一个模块中定义三维向量类,在另外一个文件中使用和测试创建的类; 1)在使用类的文件中,要求有入口函数,并分别对类的属性和方法进行结果测试; 2)创建1000个随机三维向量,要求x轴的范围在0~100,y的范围在-100~0;z的范围在-50~50,并用matplotlib把1000个向量的x坐标、y坐标和z坐标分别用三条2D曲线绘制出来(一个plot),然后给出Axis grid、 xlabel、legend、line线形等config。
时间: 2023-10-27 15:30:44 浏览: 86
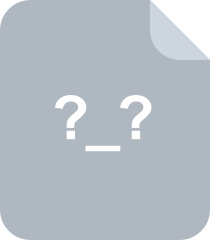
用Python自定义三维向量类,实现加减乘除等运算
好的,我来回答你的问题。
首先,关于自定义类模拟三维向量及其运算,可以按照如下代码实现:
```python
import math
class Vector3:
count = 0 # 类属性,记录实例化的对象个数
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
Vector3.count += 1 # 每次实例化对象,计数器加1
def __add__(self, other):
return Vector3(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, other):
if isinstance(other, (int, float)): # 向量与标量的乘法
return Vector3(self.x * other, self.y * other, self.z * other)
elif isinstance(other, Vector3): # 向量与向量的点积
return self.x * other.x + self.y * other.y + self.z * other.z
else:
raise ValueError("Unsupported operand type for *")
def __truediv__(self, other):
if isinstance(other, (int, float)): # 向量与标量的除法
return Vector3(self.x / other, self.y / other, self.z / other)
else:
raise ValueError("Unsupported operand type for /")
def length(self):
return math.sqrt(self.x ** 2 + self.y ** 2 + self.z ** 2)
def dot(self, other):
return self * other
def cross(self, other):
x = self.y * other.z - self.z * other.y
y = self.z * other.x - self.x * other.z
z = self.x * other.y - self.y * other.x
return Vector3(x, y, z)
def print(self):
print("({}, {}, {})".format(self.x, self.y, self.z))
```
在上面的代码中,我们定义了一个三维向量类`Vector3`,其中包含了向量的加减法、标量乘除法、向量点积和叉积、向量长度以及打印方法。同时,我们还定义了一个类属性`count`,用来记录实例化的对象个数。
在另一个文件中,我们可以按照如下方式测试`Vector3`类:
```python
import random
import matplotlib.pyplot as plt
from vector3 import Vector3 # 导入自定义的向量类
# 测试向量类的属性和方法
v1 = Vector3(1, 2, 3)
v2 = Vector3(4, 5, 6)
v3 = v1 + v2
v4 = v2 - v1
v5 = v1 * 2
v6 = 3 * v2
v7 = v1 / 2
v8 = v2 / 3
print("v1 + v2 = ", end=""), v3.print()
print("v2 - v1 = ", end=""), v4.print()
print("v1 * 2 = ", end=""), v5.print()
print("3 * v2 = ", end=""), v6.print()
print("v1 / 2 = ", end=""), v7.print()
print("v2 / 3 = ", end=""), v8.print()
print("|v1| = ", v1.length())
print("|v2| = ", v2.length())
print("v1 . v2 = ", v1.dot(v2))
print("v1 x v2 = ", end=""), v1.cross(v2).print()
print("Number of Vector3 instances: ", Vector3.count)
# 创建1000个随机三维向量并绘制2D曲线
x, y, z = [], [], []
for i in range(1000):
x.append(random.uniform(0, 100))
y.append(random.uniform(-100, 0))
z.append(random.uniform(-50, 50))
fig = plt.figure()
ax = fig.add_subplot(111)
ax.plot(x, label="x")
ax.plot(y, label="y")
ax.plot(z, label="z")
ax.grid(True)
ax.set_xlabel("Index")
ax.legend()
plt.show()
```
在上面的代码中,我们首先导入了自定义的向量类`Vector3`,然后创建了几个向量并测试了它们的加减法、标量乘除法、向量点积和叉积、向量长度以及打印方法等属性和方法。最后,我们创建了1000个随机向量,并用matplotlib将它们的x、y、z坐标分别用三条2D曲线绘制出来,并对图形进行了一些配置,如添加Axis grid、xlabel、legend和line线形等。
阅读全文
相关推荐
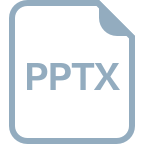
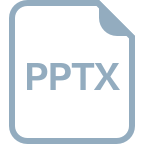
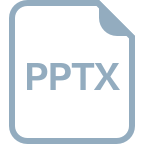
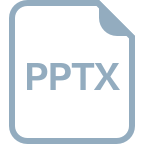
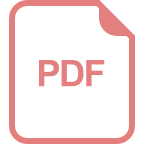
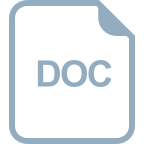
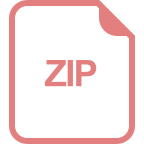
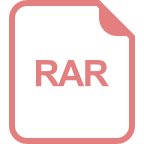
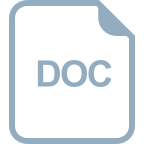
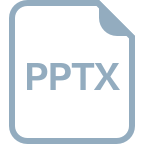
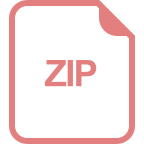
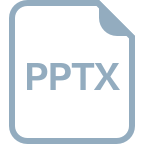
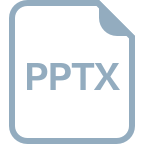
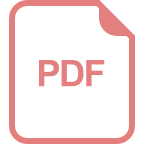