这一段Vba代码,实现根据工作表sheet1的数据,填写工作表sheet2中的数据,sheet1明细表第一列为单位名称,第二列为项目名称,第三列为项目费用明细,sheet2为统计表第一列为单位名称,第二列为费用明细(将同一单位的文本汇总到一起)
时间: 2023-09-10 07:05:34 浏览: 54
### 回答1:
Sub FillSheet2()
Dim ws1 As Worksheet
Dim ws2 As Worksheet
Dim dict As Object
Dim i As Long
Dim lastRow As Long
Dim unitName As String
Dim costDetail As String
Set ws1 = ThisWorkbook.Worksheets("Sheet1")
Set ws2 = ThisWorkbook.Worksheets("Sheet2")
Set dict = CreateObject("Scripting.Dictionary")
lastRow = ws1.Cells(ws1.Rows.Count, "A").End(xlUp).Row
For i = 2 To lastRow
unitName = ws1.Cells(i, 1).Value
costDetail = ws1.Cells(i, 3).Value
If dict.Exists(unitName) Then
dict(unitName) = dict(unitName) & ", " & costDetail
Else
dict.Add unitName, costDetail
End If
Next i
ws2.Cells.ClearContents
ws2.Cells(1, 1).Value = "单位名称"
ws2.Cells(1, 2).Value = "费用明细"
For i = 0 To dict.Count - 1
ws2.Cells(i + 2, 1).Value = dict.Keys()(i)
ws2.Cells(i + 2, 2).Value = dict.Items()(i)
Next i
End Sub
### 回答2:
利用VBA代码实现根据工作表sheet1的数据填写工作表sheet2中的数据,将同一单位的文本汇总到一起的步骤如下:
1. 在VBA编辑器中打开相应的Excel文件。
2. 在VBA编辑器中,找到工作表对象并将其分别命名为sheet1和sheet2。
3. 声明必要的变量,如单位名称(unitName)、项目名称(projectName)和项目费用明细(costDetail)。
4. 遍历sheet1的每一行数据,从第2行开始,直至最后一行。
5. 在循环中,使用unitName变量存储当前行的单位名称,使用costDetail变量存储当前行的项目费用明细。
6. 在sheet2中寻找是否有相同的单位名称。若找到,则将项目费用明细追加到相应的费用明细单元格中;若未找到,则在sheet2的下一行中填写单位名称和项目费用明细。
下面是具体的VBA代码示例:
Sub 汇总数据()
Dim sheet1 As Worksheet
Dim sheet2 As Worksheet
Set sheet1 = ThisWorkbook.Sheets("sheet1") '将"sheet1"替换为实际的工作表名称
Set sheet2 = ThisWorkbook.Sheets("sheet2") '将"sheet2"替换为实际的工作表名称
Dim unitName As String
Dim projectName As String
Dim costDetail As String
Dim lastRow As Long '记录sheet2的最后一行
lastRow = sheet2.Cells(Rows.Count, 1).End(xlUp).Row '获取sheet2的最后一行
For i = 2 To sheet1.Cells(Rows.Count, 1).End(xlUp).Row '遍历sheet1的每一行,从第2行开始
unitName = sheet1.Cells(i, 1).Value '获取单位名称
projectName = sheet1.Cells(i, 2).Value '获取项目名称
costDetail = sheet1.Cells(i, 3).Value '获取项目费用明细
If Not IsEmpty(sheet2.Cells(1, 1).Value) Then '判断sheet2第一行是否为空
If sheet2.Cells(lastRow, 1).Value = unitName Then '判断sheet2最后一行的单位名称是否与当前行的单位名称相同
sheet2.Cells(lastRow, 2).Value = sheet2.Cells(lastRow, 2).Value & vbCrLf & costDetail '如果相同,则将项目费用明细追加到相应的费用明细单元格中
Else
lastRow = lastRow + 1 '如果不同,则在sheet2的下一行写入单位名称和项目费用明细
sheet2.Cells(lastRow, 1).Value = unitName
sheet2.Cells(lastRow, 2).Value = costDetail
End If
Else
sheet2.Cells(lastRow, 1).Value = unitName '如果sheet2第一行为空,则直接在第一行填写单位名称和项目费用明细
sheet2.Cells(lastRow, 2).Value = costDetail
End If
Next i
MsgBox "数据已成功汇总到sheet2中。"
End Sub
以上代码可以根据sheet1的数据,将同一单位的费用明细汇总到sheet2中。请将代码复制到VBA编辑器中,并根据实际情况修改工作表名称。
### 回答3:
下面是一个实现根据工作表sheet1的数据,填写工作表sheet2中的数据的VBA代码。该代码将根据单位名称将费用明细汇总到统计表中。
```vba
Sub 汇总费用明细()
Dim ws1 As Worksheet, ws2 As Worksheet
Dim lastRow As Long, i As Long, j As Long
Dim unit As String, detail As String
' 设置工作表对象
Set ws1 = ThisWorkbook.Sheets("Sheet1")
Set ws2 = ThisWorkbook.Sheets("Sheet2")
' 清空统计表中的数据
ws2.Cells.ClearContents
' 获取明细表的最后一行
lastRow = ws1.Cells(ws1.Rows.Count, 1).End(xlUp).Row
' 循环遍历明细表数据
For i = 2 To lastRow
' 获取单位名称和费用明细
unit = ws1.Cells(i, 1).Value
detail = ws1.Cells(i, 3).Value
' 在统计表中查找对应的单位名称
For j = 2 To ws2.Cells(ws2.Rows.Count, 1).End(xlUp).Row
If ws2.Cells(j, 1).Value = unit Then
' 如果找到对应的单位名称,则在已存在的费用明细后面添加新的费用明细
ws2.Cells(j, 2).Value = ws2.Cells(j, 2).Value & ", " & detail
Exit For
End If
Next j
' 如果在统计表中找不到对应的单位名称,则在最后一行添加新的单位名称和费用明细
If j > ws2.Cells(ws2.Rows.Count, 1).End(xlUp).Row Then
ws2.Cells(j, 1).Value = unit
ws2.Cells(j, 2).Value = detail
End If
Next i
End Sub
```
通过运行上述代码,将会根据工作表sheet1中的数据填写工作表sheet2中的数据。在sheet2中,统计表的第一列将包含单位名称,第二列将包含该单位的所有费用明细,将同一单位的费用明细汇总到一起。
相关推荐
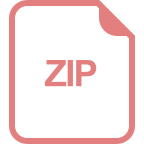
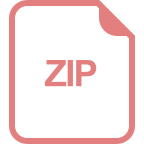














