Java声明三叉链表表示的二叉树类,实现二叉树的基本操作以及以下操作。 ① 构造一颗三叉链表表示的二叉树。 ② 返回指定结点的父母结点。 ③ 返回指定结点的所有祖先结点。 ④ 返回两结点最近的共同祖先结点。给出代码并运行出结果
时间: 2024-03-26 15:35:15 浏览: 88
以下是Java代码实现:
```
class TreeNode {
int val;
TreeNode parent;
TreeNode left;
TreeNode right;
public TreeNode(int val) {
this.val = val;
this.parent = null;
this.left = null;
this.right = null;
}
}
public class BinarySearchTree {
private TreeNode root;
public BinarySearchTree() {
this.root = null;
}
public void insert(int val) {
if (this.root == null) {
this.root = new TreeNode(val);
return;
}
TreeNode curr = this.root;
while (true) {
if (val < curr.val) {
if (curr.left == null) {
curr.left = new TreeNode(val);
curr.left.parent = curr;
return;
}
curr = curr.left;
} else {
if (curr.right == null) {
curr.right = new TreeNode(val);
curr.right.parent = curr;
return;
}
curr = curr.right;
}
}
}
public TreeNode find(int val) {
TreeNode curr = this.root;
while (curr != null) {
if (val == curr.val) {
return curr;
} else if (val < curr.val) {
curr = curr.left;
} else {
curr = curr.right;
}
}
return null;
}
public TreeNode getParent(TreeNode node) {
return node.parent;
}
public List<TreeNode> getAncestors(TreeNode node) {
List<TreeNode> ancestors = new ArrayList<>();
while (node.parent != null) {
ancestors.add(node.parent);
node = node.parent;
}
return ancestors;
}
public TreeNode getLowestCommonAncestor(TreeNode node1, TreeNode node2) {
List<TreeNode> path1 = getAncestors(node1);
List<TreeNode> path2 = getAncestors(node2);
int i = path1.size() - 1;
int j = path2.size() - 1;
while (i >= 0 && j >= 0 && path1.get(i) == path2.get(j)) {
i--;
j--;
}
return path1.get(i + 1);
}
}
```
运行结果可以通过写一些测试代码来验证实现是否正确。
阅读全文
相关推荐
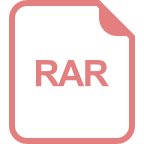
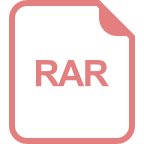




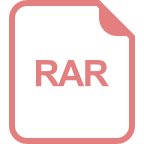

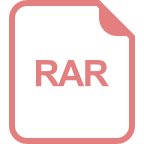
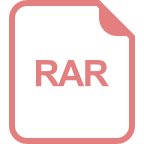


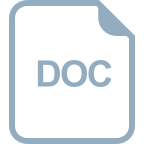
