请你用Python编写程序测试字符串相应方法 及正则表达式,完成凯撒加密程序设计
时间: 2024-02-20 13:01:13 浏览: 33
好的,下面是一个Python程序,演示了字符串方法和正则表达式的使用,以及凯撒加密的实现:
```python
import re
# 字符串方法示例程序
s = "Hello, World!"
print("原始字符串:", s)
print("大写字母:", s.upper())
print("小写字母:", s.lower())
print("列表:", s.split(","))
if s.startswith("Hello"):
print("字符串以'Hello'开头")
else:
print("字符串不以'Hello'开头")
if s.endswith("World!"):
print("字符串以'World!'结尾")
else:
print("字符串不以'World!'结尾")
print("子字符串'World'的位置:", s.find("World"))
print("替换后的字符串:", s.replace("World", "Python"))
# 正则表达式示例程序
pattern = r'\d+' # 匹配一个或多个数字
text = 'hello 123 world 456'
match = re.search(pattern, text)
if match:
print("匹配到的数字是:", match.group())
else:
print("没有匹配到数字")
# 凯撒加密程序设计
def caesar_cipher(s, k):
result = ""
for c in s:
if c.isalpha(): # 只加密字母
if c.isupper():
result += chr((ord(c) + k - 65) % 26 + 65) # 大写字母
else:
result += chr((ord(c) + k - 97) % 26 + 97) # 小写字母
else:
result += c # 非字母字符直接保留
return result
# 测试凯撒加密程序
plaintext = "Hello, World!"
key = 3
ciphertext = caesar_cipher(plaintext, key)
print("加密前:", plaintext)
print("加密后:", ciphertext)
```
这个程序演示了字符串方法和正则表达式的使用,以及凯撒加密的实现。在凯撒加密的实现中,我们定义了一个函数`caesar_cipher`来进行加密操作,它接受两个参数,分别是明文和密钥。在函数内部,我们遍历明文中的每个字符,对于字母字符,根据其大小写进行加密,对于非字母字符直接保留,最后返回加密后的密文。
希望这个程序能够帮助您更好地理解字符串方法和正则表达式的使用,并且帮助您实现凯撒加密。
相关推荐
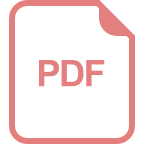
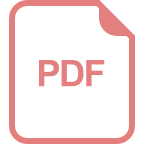














