java下载本地图片
时间: 2024-03-13 09:40:28 浏览: 68
在Java中,可以使用java.net包中的URL类和java.nio包中的Files类来下载本地图片。下面是一个简单的示例代码:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
public class ImageDownloader {
public static void main(String[] args) {
String imageUrl = "https://example.com/image.jpg"; // 图片的URL地址
String destinationPath = "C:/path/to/save/image.jpg"; // 图片保存的本地路径
try {
// 方法一:使用URL类下载图片
URL url = new URL(imageUrl);
try (InputStream in = url.openStream();
FileOutputStream out = new FileOutputStream(destinationPath)) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
}
// 方法二:使用Files类下载图片
// Path source = Paths.get(imageUrl);
// Path destination = Paths.get(destinationPath);
// Files.copy(source, destination, StandardCopyOption.REPLACE_EXISTING);
System.out.println("图片下载成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意替换`imageUrl`和`destinationPath`变量的值为你实际需要下载的图片的URL和本地保存路径。上述代码提供了两种下载图片的方法,你可以根据需要选择其中一种方法。
阅读全文
相关推荐







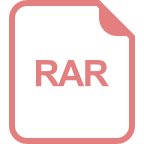





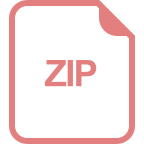



