linux多线程实验
时间: 2023-12-11 21:30:37 浏览: 175
Linux下的多线程编程遵循POSIX线程接口,也称为pthread。在Linux系统下编写多线程程序需要使用头文件pthread.h,连接时需要使用库libpthread.a。下面是一个简单的多线程编程实验步骤与操作指导:
1. 编写多线程程序example.c,包含头文件<stdio.h>,<stdlib.h>和<pthread.h>。
2. 在程序中定义一个线程函数thread,该函数输出"This is a pthread."。
3. 在主函数main中,使用pthread_create函数创建一个新线程,并将线程函数thread作为参数传递给pthread_create函数。
4. 在主函数中,使用for循环输出"This is the main process."。
5. 使用pthread_join函数等待新线程结束。
6. 编译程序,链接libpthread.a库,生成可执行文件。
7. 运行程序,观察输出结果。
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void thread(void) {
int i;
for(i=0;i<3;i++)
printf("This is a pthread.\n");
}
int main(void) {
pthread_t id;
int i,ret;
ret=pthread_create(&id,NULL,(void *) thread,NULL);
if(ret!=0) {
printf ("Create pthread error!\n");
exit (1);
}
for(i=0;i<3;i++)
printf("This is the main process.\n");
pthread_join(id,NULL);
return 0;
}
```
阅读全文
相关推荐
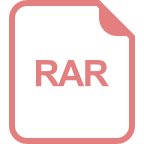
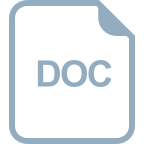
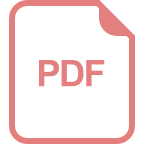

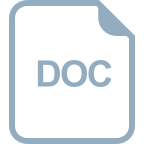
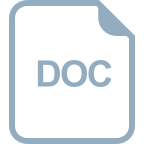
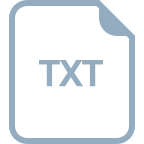
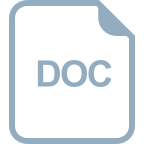
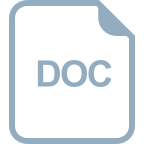
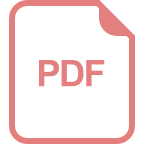
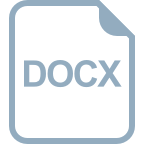
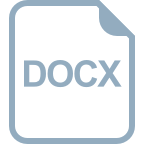
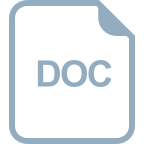
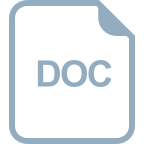
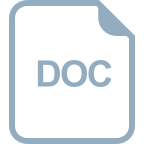
