第34讲 js超级玛丽小游戏
时间: 2023-07-08 19:05:16 浏览: 47
好的,让我们开始制作一个简单的JS超级玛丽小游戏吧!
首先,我们需要一个画布和一个可以控制的角色。我们可以使用HTML5 Canvas来创建游戏画面,然后使用JavaScript控制角色的移动。
HTML代码:
```
<canvas id="gameCanvas" width="800" height="600"></canvas>
```
接下来,我们需要一个JavaScript文件来控制游戏逻辑。我们首先定义一个Game类,它包含游戏的各种元素和方法。我们还需要定义一个角色类,它包含角色的属性和方法。
JS代码:
```
// 定义角色类
class Character {
constructor(x, y, width, height, speed) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
this.speed = speed;
}
moveLeft() {
this.x -= this.speed;
}
moveRight() {
this.x += this.speed;
}
moveUp() {
this.y -= this.speed;
}
moveDown() {
this.y += this.speed;
}
}
// 定义游戏类
class Game {
constructor(canvasId) {
this.canvas = document.getElementById(canvasId);
this.ctx = this.canvas.getContext("2d");
this.character = new Character(100, 100, 50, 50, 5);
this.keysPressed = {};
this.setupEventListeners();
}
// 监听键盘事件
setupEventListeners() {
document.addEventListener("keydown", (e) => {
this.keysPressed[e.key] = true;
});
document.addEventListener("keyup", (e) => {
delete this.keysPressed[e.key];
});
}
// 更新游戏状态
update() {
if (this.keysPressed["ArrowLeft"]) {
this.character.moveLeft();
}
if (this.keysPressed["ArrowRight"]) {
this.character.moveRight();
}
if (this.keysPressed["ArrowUp"]) {
this.character.moveUp();
}
if (this.keysPressed["ArrowDown"]) {
this.character.moveDown();
}
}
// 渲染画面
render() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height);
this.ctx.fillStyle = "blue";
this.ctx.fillRect(
this.character.x,
this.character.y,
this.character.width,
this.character.height
);
}
// 开始游戏循环
start() {
setInterval(() => {
this.update();
this.render();
}, 1000 / 60);
}
}
// 创建游戏实例并开始游戏
const game = new Game("gameCanvas");
game.start();
```
在这个代码中,我们首先定义了一个Character类和一个Game类。Character类包含角色的属性和移动方法,Game类包含游戏的各种元素和方法,包括角色、键盘事件监听、更新游戏状态、渲染画面和开始游戏循环。
在Game类的构造函数中,我们创建了一个角色实例,并设置了键盘事件监听。在update()方法中,我们根据按下的键盘键来移动角色。在render()方法中,我们清除画布并绘制角色。在start()方法中,我们使用setInterval()来循环更新游戏状态和渲染画面。
现在我们可以在网页上看到一个蓝色的方块,我们可以使用方向键来控制它的移动了。接下来,我们可以添加更多的元素和功能来完善这个小游戏,比如障碍物、积分、生命值、敌人等等。
相关推荐
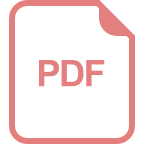
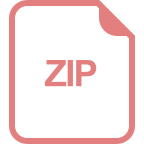
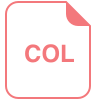
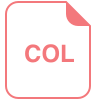
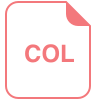
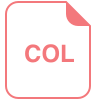
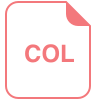









