jdbc连接数据库步骤
时间: 2023-06-19 20:09:18 浏览: 75
以下是Java程序通过JDBC连接数据库的基本步骤:
1. 导入JDBC相关的jar包。
2. 加载并注册数据库的JDBC驱动程序。
3. 创建数据库连接,使用DriverManager类的getConnection方法,传入数据库的URL、用户名和密码等参数。
4. 创建Statement对象,用于执行SQL语句。
5. 执行SQL语句,可以使用Statement类的executeQuery方法执行查询语句,使用executeUpdate方法执行更新、插入、删除等操作。
6. 处理查询结果,ResultSet类提供了获取查询结果的方法。
7. 关闭ResultSet、Statement和Connection对象,释放资源。
下面是一个示例代码,连接MySQL数据库:
```java
import java.sql.*;
public class JdbcTest {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 注册 JDBC 驱动
Class.forName("com.mysql.jdbc.Driver");
// 打开连接
System.out.println("连接数据库...");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
// 执行查询
System.out.println("实例化Statement对象...");
stmt = conn.createStatement();
String sql;
sql = "SELECT id, name, age FROM student";
rs = stmt.executeQuery(sql);
// 处理结果集
while(rs.next()){
// 通过字段检索
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
// 输出数据
System.out.print("ID: " + id);
System.out.print(", Name: " + name);
System.out.println(", Age: " + age);
}
// 完成后关闭
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
// 处理 JDBC 错误
se.printStackTrace();
} catch (Exception e) {
// 处理 Class.forName 错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if(stmt!=null) stmt.close();
} catch (SQLException se2) {
} // 什么都不做
try {
if(conn!=null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
其中,需要将`com.mysql.jdbc.Driver`替换为相应的数据库驱动程序类名,`jdbc:mysql://localhost:3306/test`替换为相应的数据库URL,`root`替换为相应的用户名,`password`替换为相应的密码。
阅读全文
相关推荐
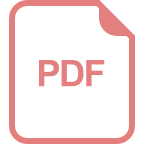
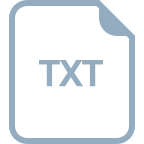
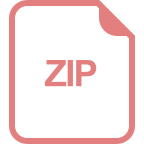
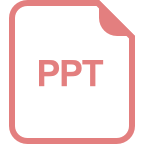
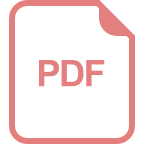
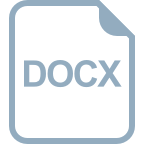
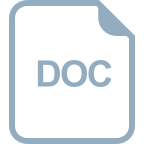
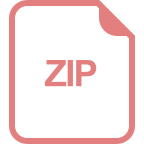
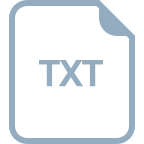
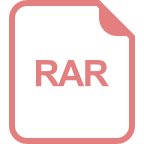
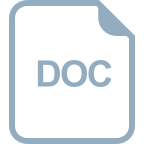