getter和setter
时间: 2023-09-29 11:08:58 浏览: 48
Getter和Setter是面向对象编程中常用的两个概念,用来访问和修改对象的属性。Getter通常用于获取对象的属性值,而Setter则用于设置对象的属性值。Getter和Setter的作用在于对属性的访问进行控制和限制,例如可以在Getter中添加一些逻辑来控制属性的访问权限,或者在Setter中进行数据验证、格式化等操作,保证属性值的正确性。在许多编程语言中,Getter和Setter通常以方法的形式实现,例如在Java中通常使用getXxx()和setXxx()方法来表示Getter和Setter。
相关问题
oc getter和setter
在Objective-C中,getter和setter是用来访问和修改对象属性的方法。它们可以手动编写,也可以使用@property关键字来自动生成。
getter方法用来获取属性的值,setter方法用来设置属性的值。默认情况下,如果你没有手动编写getter和setter方法,那么编译器会自动为你生成默认的getter和setter方法。默认的getter和setter方法会自动从对应的实例变量中获取或设置属性的值。
以下是手动编写getter和setter方法的示例:
```
@interface Person : NSObject
@property (nonatomic, strong) NSString *name;
@end
@implementation Person
- (NSString *)name {
return _name;
}
- (void)setName:(NSString *)name {
_name = name;
}
@end
```
使用@property关键字可以自动生成getter和setter方法,例如:
```
@interface Person : NSObject
@property (nonatomic, strong) NSString *name;
@end
@implementation Person
@end
```
这里并没有手动编写getter和setter方法,但编译器会自动为我们生成默认的getter和setter方法。
使用生成的getter和setter方法非常简单,例如:
```
Person *person = [[Person alloc] init];
person.name = @"Tom"; // 使用setter方法设置属性值
NSString *name = person.name; // 使用getter方法获取属性值
```
需要注意的是,如果你希望对属性进行自定义的访问和修改操作,例如进行类型转换、数据验证等操作,那么你可以手动编写getter和setter方法来实现。
getter和setter方法
getter和setter方法是用于访问和修改对象属性的方法。getter方法用于获取对象属性的值,setter方法用于设置对象属性的值。
例如,假设有一个Person类,其中有一个name属性:
```
class Person {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
```
在上面的代码中,getName()方法是一个getter方法,它返回对象的name属性的值,而setName()方法是一个setter方法,它设置对象的name属性的值。通过使用这些方法,可以在不直接访问属性的情况下对其进行访问和修改,从而提高了封装性和数据安全性。
相关推荐
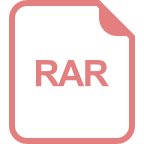
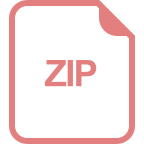
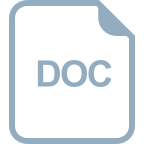
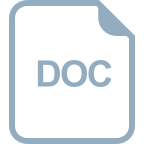
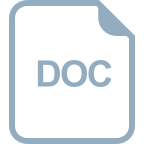
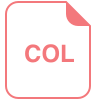








