用C语言完成打开特定目录下文件查找特定字符串并修改
时间: 2023-12-21 18:07:33 浏览: 92
可以使用以下步骤来完成用C语言打开特定目录下文件查找特定字符串并修改的任务:
1. 使用`opendir`函数打开指定目录。
2. 使用`readdir`函数遍历目录中的所有文件。
3. 对于每个文件,使用`fopen`函数打开并读取文件内容。
4. 使用`strstr`函数查找文件内容中是否包含特定字符串。
5. 如果找到特定字符串,可以使用`fseek`函数找到字符串所在的位置,然后使用`fwrite`函数将修改后的字符串写回文件中。
6. 关闭文件并继续遍历下一个文件,直到目录中的所有文件都被遍历完毕。
7. 关闭目录并结束程序。
以下是一份示例代码,可以根据具体需求进行修改:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
void search_and_replace(const char *filename, const char *search, const char *replace) {
FILE *fp = fopen(filename, "r+");
if (fp == NULL) {
printf("Failed to open file: %s\n", filename);
return;
}
char buf[1024];
size_t search_len = strlen(search);
size_t replace_len = strlen(replace);
while (fgets(buf, sizeof(buf), fp) != NULL) {
char *pos;
while ((pos = strstr(buf, search)) != NULL) {
fseek(fp, pos - buf, SEEK_CUR);
fputs(replace, fp);
memmove(pos + replace_len, pos + search_len, strlen(pos + search_len) + 1);
rewind(fp);
fseek(fp, strlen(buf) - strlen(pos + search_len), SEEK_CUR);
}
}
fclose(fp);
}
int main() {
const char *dir_path = "/path/to/directory";
const char *search_str = "hello";
const char *replace_str = "world";
DIR *dir = opendir(dir_path);
if (dir == NULL) {
printf("Failed to open directory: %s\n", dir_path);
return 1;
}
struct dirent *entry;
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG) {
char path[1024];
snprintf(path, sizeof(path), "%s/%s", dir_path, entry->d_name);
search_and_replace(path, search_str, replace_str);
}
}
closedir(dir);
return 0;
}
```
阅读全文
相关推荐
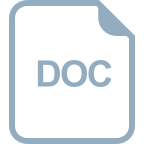
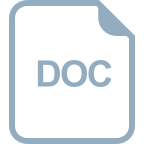
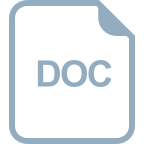







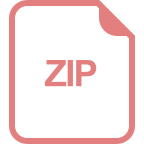
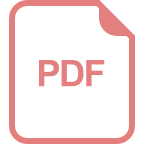
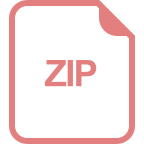





