C#窗口程序 将当前文件夹下的所有文件和文件夹打包,成abc.exe;abc.exe执行时具有如下功能: 1. 读取注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值保存为字符串strShell,strShell的字符串格式举例为"C:\2.4.7460.12546\autostart.exe doctorold.exe"; 2. 将strShell识别字符串中路径的子字符串保存为strPath; 3. 读取当前目录下的DoctorOld.exe文件版本保存为字符串strNewVer; 4. 新建"C:\"+strNewVer文件夹,其中包括判断是否存在,进行提示“版本相同是否进行强制更新”,如果强制更新则将旧文件夹进行重命名,重命名规则为在文件夹后加当前时间,将新文件夹路径给strPath赋值; 5. 复制当前文件夹所有文件至"C:\"+strNewVer文件夹; 6. 复制strPath目录下的asd.txt文件至"C:\"+strNewVer文件夹直接覆盖; 7. 查找strShell中是否含有“Doctorold.exe”、"Q.exe"、"W.exe",分别存布尔型变量; 8. 如果含有“Doctorold.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe doctorold.exe"; 9. 如果含有“Q.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe Q.exe"; 10. 如果含有“W.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe W.exe";
时间: 2023-07-05 15:05:27 浏览: 71
以下是C#窗口程序的代码实现:
```csharp
using System;
using System.IO;
using System.Diagnostics;
using Microsoft.Win32;
namespace FilePacker
{
class Program
{
static void Main(string[] args)
{
// 1. 读取注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值保存为字符串strShell
string strShell = "";
RegistryKey key = Registry.LocalMachine.OpenSubKey(@"System\Setup");
if (key != null)
{
strShell = key.GetValue("shell") as string;
key.Close();
}
// 2. 将strShell识别字符串中路径的子字符串保存为strPath
string[] shellItems = strShell.Split(' ');
string strPath = "";
foreach (var item in shellItems)
{
if (item.EndsWith(".exe"))
{
strPath = Path.GetDirectoryName(item);
break;
}
}
// 3. 读取当前目录下的DoctorOld.exe文件版本保存为字符串strNewVer
string doctorOldPath = Path.Combine(Environment.CurrentDirectory, "DoctorOld.exe");
string strNewVer = FileVersionInfo.GetVersionInfo(doctorOldPath).FileVersion;
// 4. 新建"C:\"+strNewVer文件夹,其中包括判断是否存在,进行提示“版本相同是否进行强制更新”,如果强制更新则将旧文件夹进行重命名,重命名规则为在文件夹后加当前时间,将新文件夹路径给strPath赋值
string newPath = Path.Combine("C:\\", strNewVer);
if (Directory.Exists(newPath))
{
Console.WriteLine("版本相同是否进行强制更新?(y/n)");
if (Console.ReadLine() == "y")
{
string renamedPath = newPath + DateTime.Now.ToString("yyyyMMddHHmmss");
Directory.Move(newPath, renamedPath);
}
else
{
return;
}
}
Directory.CreateDirectory(newPath);
strPath = newPath;
// 5. 复制当前文件夹所有文件至"C:\"+strNewVer文件夹
foreach (string file in Directory.GetFiles(Environment.CurrentDirectory))
{
File.Copy(file, Path.Combine(newPath, Path.GetFileName(file)), true);
}
foreach (string dir in Directory.GetDirectories(Environment.CurrentDirectory))
{
string newDir = Path.Combine(newPath, Path.GetFileName(dir));
Directory.CreateDirectory(newDir);
foreach (string file in Directory.GetFiles(dir))
{
File.Copy(file, Path.Combine(newDir, Path.GetFileName(file)), true);
}
}
// 6. 复制strPath目录下的asd.txt文件至"C:\"+strNewVer文件夹直接覆盖
string asdPath = Path.Combine(strPath, "asd.txt");
if (File.Exists(asdPath))
{
File.Copy(asdPath, Path.Combine(newPath, "asd.txt"), true);
}
// 7. 查找strShell中是否含有“Doctorold.exe”、"Q.exe"、"W.exe",分别存布尔型变量
bool hasDoctorOld = false;
bool hasQ = false;
bool hasW = false;
foreach (var item in shellItems)
{
if (item.EndsWith("Doctorold.exe"))
{
hasDoctorOld = true;
}
if (item.EndsWith("Q.exe"))
{
hasQ = true;
}
if (item.EndsWith("W.exe"))
{
hasW = true;
}
}
// 8. 如果含有“Doctorold.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe doctorold.exe";
if (hasDoctorOld)
{
strShell = strShell.Replace("Doctorold.exe", Path.Combine(newPath, "autostart.exe doctorold.exe"));
}
// 9. 如果含有“Q.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe Q.exe";
if (hasQ)
{
strShell = strShell.Replace("Q.exe", Path.Combine(newPath, "autostart.exe Q.exe"));
}
// 10. 如果含有“W.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe W.exe";
if (hasW)
{
strShell = strShell.Replace("W.exe", Path.Combine(newPath, "autostart.exe W.exe"));
}
// 更新注册表
key = Registry.LocalMachine.OpenSubKey(@"System\Setup", true);
if (key != null)
{
key.SetValue("shell", strShell);
key.Close();
}
Console.WriteLine("打包完成,按任意键退出...");
Console.ReadKey();
}
}
}
```
相关推荐
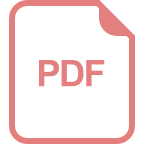














