巴特沃兹滤波C++代码
时间: 2023-10-20 09:08:06 浏览: 87
以下是一个简单的巴特沃兹滤波器的C代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979323846
// 巴特沃兹滤波器
void butterworth_filter(double *input, double *output, int n, double wc, int order)
{
double *a, *b, *x, *y;
double w, alpha, beta, tmp;
int i, j;
// 分配内存
a = (double *)malloc(sizeof(double) * (order + 1));
b = (double *)malloc(sizeof(double) * (order + 1));
x = (double *)malloc(sizeof(double) * (order + 1));
y = (double *)malloc(sizeof(double) * (order + 1));
// 计算系数
w = 2 * PI * wc;
alpha = sin(w) / (2 * order);
beta = cos(w);
for (i = 0; i <= order; i++) {
tmp = sin((2 * i + 1) * PI / (4 * order));
a[i] = -2 * beta * tmp;
b[i] = alpha + beta * tmp;
}
// 滤波
for (i = 0; i < n; i++) {
x[0] = input[i];
y[0] = b[0] * x[0];
for (j = 1; j <= order; j++) {
x[j] = x[j-1];
y[j] = b[j] * x[j] + a[j] * y[j-1];
}
output[i] = y[order];
}
// 释放内存
free(a);
free(b);
free(x);
free(y);
}
// 测试
int main()
{
double input[] = { 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0 };
double output[9];
int i;
butterworth_filter(input, output, 9, 0.4, 4);
for (i = 0; i < 9; i++) {
printf("%f\n", output[i]);
}
return 0;
}
注意:上述代码仅供参考,实际应用中需要根据具体需求进行修改。
阅读全文
相关推荐








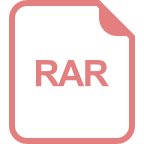


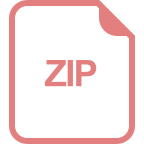






