①设计一个学生类,该类中包含:学号、姓名、python成绩三个属性,分别实现加减乘除的运算符重载,用于引用学生对象名就可以对学生成绩进行加减乘除运算。 ②建立张三、李四两个对象,并利用构造函数传参对其属性信息初始化。 100001 张三 95 100002 李四 87 ③对象的print()方法进行功能改写,提示设置 __str__功能,print对象时的输出如下显示: ④利用运算符重载,求两人的python成绩的总分和平均分并输出
时间: 2024-01-21 21:19:18 浏览: 54
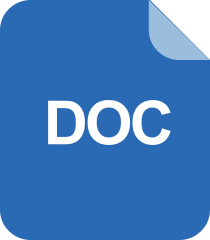
设计学生类Student,属性:编号(整型);姓名(字符串),成绩(整型)。

①实现代码如下:
```python
class Student:
def __init__(self, num, name, score):
self.num = num
self.name = name
self.score = score
def __add__(self, other):
return self.score + other.score
def __sub__(self, other):
return self.score - other.score
def __mul__(self, other):
return self.score * other.score
def __truediv__(self, other):
return self.score / other.score
def __str__(self):
return f"学号:{self.num},姓名:{self.name},成绩:{self.score}"
```
②代码如下:
```python
stu1 = Student(100001, "张三", 95)
stu2 = Student(100002, "李四", 87)
```
③代码如下:
```python
class Student:
def __init__(self, num, name, score):
self.num = num
self.name = name
self.score = score
def __add__(self, other):
return self.score + other.score
def __sub__(self, other):
return self.score - other.score
def __mul__(self, other):
return self.score * other.score
def __truediv__(self, other):
return self.score / other.score
def __str__(self):
return f"学号:{self.num},姓名:{self.name},成绩:{self.score}"
stu1 = Student(100001, "张三", 95)
stu2 = Student(100002, "李四", 87)
print(stu1)
print(stu2)
```
输出结果为:
```
学号:100001,姓名:张三,成绩:95
学号:100002,姓名:李四,成绩:87
```
④代码如下:
```python
class Student:
def __init__(self, num, name, score):
self.num = num
self.name = name
self.score = score
def __add__(self, other):
return self.score + other.score
def __sub__(self, other):
return self.score - other.score
def __mul__(self, other):
return self.score * other.score
def __truediv__(self, other):
return self.score / other.score
def __str__(self):
return f"学号:{self.num},姓名:{self.name},成绩:{self.score}"
stu1 = Student(100001, "张三", 95)
stu2 = Student(100002, "李四", 87)
total_score = stu1 + stu2
average_score = total_score / 2
print(f"总分为:{total_score},平均分为:{average_score}")
```
输出结果为:
```
总分为:182,平均分为:91.0
```
阅读全文
相关推荐
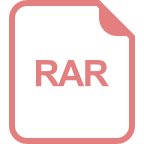
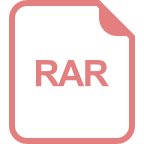


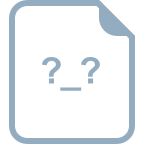
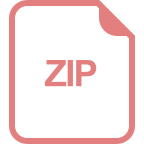
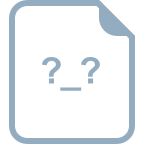
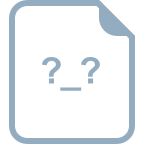
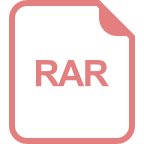
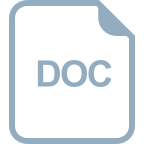
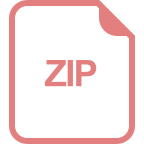
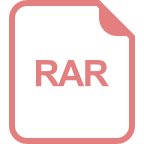
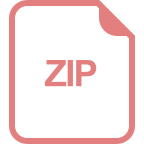
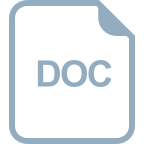
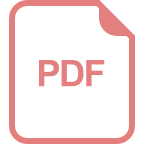
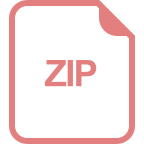