用Java实现在窗体中编写一个图形界面程序,窗体中安排一个文本框,一个按钮和一个标签. 在文本中输入任意一个加法表达式(例如: 23+563+48+82) 点击按钮,则将表达式的计算结果显示在标签中
时间: 2023-06-03 07:07:54 浏览: 247
好的,这是一个关于Java编程的问题,可以回答。以下是实现该程序的基本步骤:
1. 创建一个窗体,并在其中安排一个文本框、一个按钮和一个标签。
2. 给按钮添加一个事件监听器,当用户点击按钮时触发该监听器。
3. 在监听器中获取文本框中的加法表达式,使用Java的计算方法计算出结果。
4. 将计算结果显示在标签中。
代码示例:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Calculator extends JFrame {
JTextField textBox;
JButton calculateButton;
JLabel resultLabel;
public Calculator() {
textBox = new JTextField(20);
calculateButton = new JButton("Calculate");
calculateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String expression = textBox.getText();
int result = calculateExpression(expression);
resultLabel.setText(String.valueOf(result));
}
});
resultLabel = new JLabel("");
JPanel panel = new JPanel();
panel.add(textBox);
panel.add(calculateButton);
panel.add(resultLabel);
add(panel);
setTitle("Calculator");
setSize(300, 100);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private int calculateExpression(String expression) {
String[] operands = expression.split(" ");
int result = Integer.parseInt(operands[0]);
for (int i = 1; i < operands.length; i++) {
int operand = Integer.parseInt(operands[i]);
result += operand;
}
return result;
}
public static void main(String[] args) {
new Calculator();
}
}
```
在这个程序中,我们使用了Java的Swing库来创建窗体和界面组件,实现了上述的基本步骤。注意,在事件监听器中获取到的文本框中的内容是一个字符串,需要使用Java的字符串处理方法将其解析为数字进行计算,最后将结果以字符串形式显示在标签中。
阅读全文
相关推荐






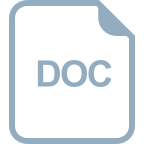








