使用.net 6 封装一个数据库仓储基类
时间: 2024-05-03 08:17:29 浏览: 18
好的,以下是一个使用 .NET 6 的基于泛型的数据库仓储基类的示例:
```csharp
using Microsoft.EntityFrameworkCore;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Threading.Tasks;
namespace YourNamespace
{
public class Repository<T> : IRepository<T> where T : class
{
private readonly DbContext _dbContext;
private readonly DbSet<T> _dbSet;
public Repository(DbContext dbContext)
{
_dbContext = dbContext;
_dbSet = _dbContext.Set<T>();
}
public async Task<IEnumerable<T>> GetAllAsync()
{
return await _dbSet.ToListAsync();
}
public async Task<IEnumerable<T>> FindAsync(Expression<Func<T, bool>> predicate)
{
return await _dbSet.Where(predicate).ToListAsync();
}
public async Task<T> GetByIdAsync(int id)
{
return await _dbSet.FindAsync(id);
}
public async Task AddAsync(T entity)
{
await _dbSet.AddAsync(entity);
}
public void Update(T entity)
{
_dbSet.Update(entity);
}
public void Remove(T entity)
{
_dbSet.Remove(entity);
}
public async Task<int> SaveChangesAsync()
{
return await _dbContext.SaveChangesAsync();
}
}
public interface IRepository<T> where T : class
{
Task<IEnumerable<T>> GetAllAsync();
Task<IEnumerable<T>> FindAsync(Expression<Func<T, bool>> predicate);
Task<T> GetByIdAsync(int id);
Task AddAsync(T entity);
void Update(T entity);
void Remove(T entity);
Task<int> SaveChangesAsync();
}
}
```
使用方法:
1. 首先需要引入依赖注入
```csharp
services.AddDbContext<YourDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("YourConnectionString")));
services.AddScoped(typeof(IRepository<>), typeof(Repository<>));
```
2. 在需要用到仓储的类中注入 IRepository
```csharp
private readonly IRepository<YourEntity> _yourEntityRepository;
public YourClass(IRepository<YourEntity> yourEntityRepository)
{
_yourEntityRepository = yourEntityRepository;
}
```
3. 使用仓储方法进行数据操作
```csharp
var allEntities = await _yourEntityRepository.GetAllAsync();
var entity = await _yourEntityRepository.GetByIdAsync(1);
await _yourEntityRepository.AddAsync(newEntity);
await _yourEntityRepository.SaveChangesAsync();
entity.Name = "New Name";
_yourEntityRepository.Update(entity);
await _yourEntityRepository.SaveChangesAsync();
_yourEntityRepository.Remove(entity);
await _yourEntityRepository.SaveChangesAsync();
```
希望这个示例能够帮助到你!
相关推荐
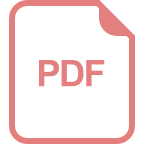
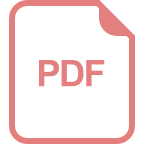














