c语言设计一个子函数,统计任意一串字符中数字字符的个数
时间: 2024-05-06 20:17:14 浏览: 95
可以使用以下代码来实现统计数字字符个数的功能:
```c
#include <stdio.h>
int count_digits(char* str) {
int count = 0;
for(int i=0; str[i]!='\0'; i++) {
if(str[i]>='0' && str[i]<='9') {
count++;
}
}
return count;
}
int main() {
char str[100];
printf("请输入一串字符:");
scanf("%s", str);
int digits = count_digits(str);
printf("数字字符的个数为:%d\n", digits);
return 0;
}
```
在上面的代码中,`count_digits` 函数接收一个字符串作为参数,遍历字符串中的每个字符,如果该字符是数字字符,则将计数器加 1。最后返回计数器的值。
在 `main` 函数中,首先让用户输入一串字符,然后调用 `count_digits` 函数来统计其中的数字字符个数,并输出结果。
相关问题
c语言 设计一个子函数,统计任意一串字符中数字字符的个数,并在主函数中调用此函数
实现如下:
```c
#include <stdio.h>
int count_digits(char *str) {
int count = 0;
while (*str != '\0') {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
int main() {
char str[100];
printf("请输入任意一串字符:");
fgets(str, 100, stdin);
int count = count_digits(str);
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
这段代码中,`count_digits` 函数用来统计字符串中数字字符的个数。在主函数中,我们先输入一串字符,然后调用 `count_digits` 函数进行统计,并输出结果。
c语言 设计一个子函数,统计任意一串字符中数字字符的个数,并在主函数中调用此函数。
以下是一个统计数字字符个数的示例代码:
```c
#include <stdio.h>
int count_digits(char *str) {
int count = 0;
while (*str != '\0') {
if (*str >= '0' && *str <= '9') { // 如果当前字符是数字字符
count++; // 数字字符计数器加1
}
str++; // 继续遍历下一个字符
}
return count;
}
int main() {
char str[100];
printf("请输入一串字符:");
gets(str);
int digits = count_digits(str);
printf("数字字符的个数为:%d\n", digits);
return 0;
}
```
在上述代码中,`count_digits()` 函数用于统计任意一串字符中数字字符的个数。它接受一个指向字符数组的指针作为参数,然后遍历整个字符串,对每个字符进行判断,如果当前字符是数字字符,则将计数器加1。最后返回计数器的值。
在 `main()` 函数中,首先提示用户输入一串字符,然后调用 `count_digits()` 函数统计数字字符的个数,并输出结果。注意,这里使用了不安全的 `gets()` 函数读取字符串,实际代码中应该使用更加安全的函数替代。
阅读全文
相关推荐
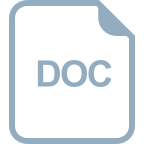













