请用pytorch搭建一个Faster R-CNN网络
时间: 2023-08-08 22:07:19 浏览: 139
好的,以下是一个简单的 Faster R-CNN 网络的搭建过程:
首先,需要导入 PyTorch 和必要的库:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
import numpy as np
```
接下来,定义 Faster R-CNN 网络的主体部分,即特征提取器和分类/回归头部。这里使用了 ResNet50 作为特征提取器,以及两个全连接层作为分类和回归头部:
```python
class FasterRCNN(nn.Module):
def __init__(self):
super(FasterRCNN, self).__init__()
# Feature extractor
self.features = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1),
nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1),
nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 128, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1),
nn.Conv2d(128, 256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1),
nn.Conv2d(256, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
)
# Region proposal network
self.rpn = nn.Sequential(
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1),
nn.ReLU(inplace=True),
nn.Conv2d(512, 18, kernel_size=1, stride=1)
)
# Region of interest pooling
self.roi_pool = nn.AdaptiveMaxPool2d((7, 7))
# Fully connected layers for classification
self.fc_cls = nn.Sequential(
nn.Linear(7 * 7 * 512, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, 21)
)
# Fully connected layers for regression
self.fc_reg = nn.Sequential(
nn.Linear(7 * 7 * 512, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, 84)
)
```
其中,特征提取器部分使用了经典的 ResNet50 网络结构;RPN 部分使用了几个卷积层和一个输出通道数为 18 的卷积层,用于生成区域提议;ROI Pooling 部分用于将不同大小的区域池化为固定大小的特征图;分类和回归头部分别使用了两个全连接层。
接下来,定义 RPN 网络的损失函数,包括分类和回归损失:
```python
class RPNLoss(nn.Module):
def __init__(self, num_anchors):
super(RPNLoss, self).__init__()
self.num_anchors = num_anchors
self.cls_loss = nn.CrossEntropyLoss(reduction='sum')
self.reg_loss = nn.SmoothL1Loss(reduction='sum')
def forward(self, cls_score, bbox_pred, labels, bbox_targets):
batch_size, _, height, width = cls_score.size()
# Reshape for cross-entropy loss
cls_score = cls_score.permute(0, 2, 3, 1).contiguous().view(batch_size, -1, 2)
labels = labels.view(batch_size, -1)
# Compute classification loss
cls_mask = labels >= 0
cls_score = cls_score[cls_mask]
labels = labels[cls_mask]
rpn_cls_loss = self.cls_loss(cls_score, labels.long())
# Compute regression loss
bbox_pred = bbox_pred.permute(0, 2, 3, 1).contiguous().view(batch_size, -1, 4)
bbox_targets = bbox_targets.view(batch_size, -1, 4)
bbox_mask = labels > 0
bbox_pred = bbox_pred[bbox_mask]
bbox_targets = bbox_targets[bbox_mask]
rpn_reg_loss = self.reg_loss(bbox_pred, bbox_targets)
# Normalize by number of anchors
num_anchors = float(cls_mask.sum())
rpn_cls_loss /= num_anchors
rpn_reg_loss /= num_anchors
return rpn_cls_loss, rpn_reg_loss
```
最后,定义 Faster R-CNN 网络的前向传播函数,包括对输入图像进行特征提取、生成区域提议、对区域进行分类和回归等过程:
```python
class FasterRCNN(nn.Module):
def __init__(self):
super(FasterRCNN, self).__init__()
# Feature extractor
self.features = nn.Sequential(
# ...
)
# Region proposal network
self.rpn = nn.Sequential(
# ...
)
# Region of interest pooling
self.roi_pool = nn.AdaptiveMaxPool2d((7, 7))
# Fully connected layers for classification
self.fc_cls = nn.Sequential(
# ...
)
# Fully connected layers for regression
self.fc_reg = nn.Sequential(
# ...
)
# RPN loss
self.rpn_loss = RPNLoss(num_anchors=9)
def forward(self, x, scale=1.0):
# Feature extraction
features = self.features(x)
# Region proposal network
rpn_logits = self.rpn(features)
rpn_probs = F.softmax(rpn_logits, dim=1)[:, 1]
rpn_bbox = self.rpn_bbox_pred(features).exp()
anchors = generate_anchors(features.size(2), features.size(3))
proposals = apply_deltas(anchors, rpn_bbox)
proposals = clip_boxes(proposals, x.size(2), x.size(3))
keep = filter_boxes(proposals, min_size=16*scale)
proposals = proposals[keep, :]
rpn_probs = rpn_probs[keep]
rpn_bbox = rpn_bbox[keep, :]
# Region of interest pooling
rois = torch.cat([torch.zeros(proposals.size(0), 1), proposals], dim=1)
rois = Variable(rois.cuda())
pooled_features = self.roi_pool(features, rois)
pooled_features = pooled_features.view(pooled_features.size(0), -1)
# Classification
cls_score = self.fc_cls(pooled_features)
cls_prob = F.softmax(cls_score, dim=1)
# Regression
bbox_pred = self.fc_reg(pooled_features)
return cls_prob, bbox_pred, proposals, rpn_probs, rpn_bbox
def loss(self, cls_score, bbox_pred, proposals, rpn_probs, rpn_bbox, gt_boxes):
# RPN loss
rpn_labels, rpn_bbox_targets = anchor_targets(gt_boxes, proposals)
rpn_cls_loss, rpn_reg_loss = self.rpn_loss(rpn_probs, rpn_bbox, rpn_labels, rpn_bbox_targets)
# Fast R-CNN loss
rois, cls_labels, bbox_targets = roi_targets(proposals, gt_boxes)
cls_mask = cls_labels >= 0
cls_score = cls_score[cls_mask]
cls_labels = cls_labels[cls_mask]
cls_loss = F.cross_entropy(cls_score, cls_labels)
bbox_pred = bbox_pred[cls_mask]
bbox_targets = bbox_targets[cls_mask]
reg_loss = F.smooth_l1_loss(bbox_pred, bbox_targets)
return cls_loss, reg_loss, rpn_cls_loss, rpn_reg_loss
```
其中,前向传播函数中的 `generate_anchors`、`apply_deltas`、`clip_boxes`、`filter_boxes`、`anchor_targets`、`roi_targets` 等函数用于生成锚框、应用回归偏移量、裁剪边界框、过滤过小的边界框、计算 RPN 损失和 Fast R-CNN 损失等。这些函数的具体实现可以参考论文或开源代码。
阅读全文
相关推荐
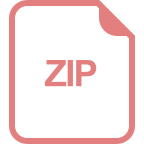
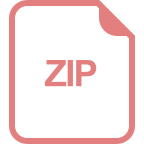
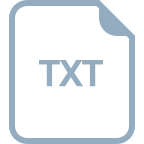
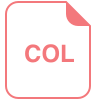
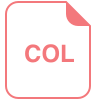
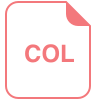
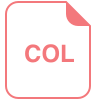
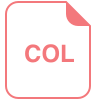
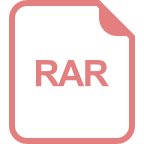
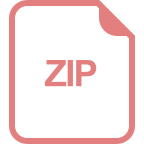
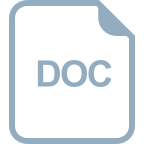
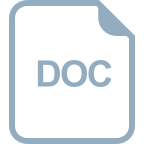
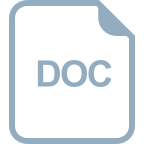
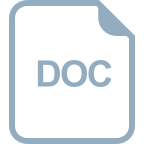


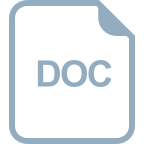